The Python type()
function is an extremely useful tool for determining the type of an object. Developers can easily check what type a variable or object is, and use this information for conditional processing or debugging. In this post, we will explore the basic usage of the type()
function as well as various ways it can be applied.
Table of Contents
Basic Concept of Python type()
Function
The type()
function is a built-in Python function that returns the type of the object passed as an argument.
num = 23
print(type(num))
In the code above, the value assigned to the variable num
is 23
, which is an integer. Therefore, type(num)
outputs <class 'int'>
.
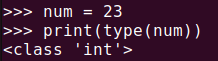
type()
on an integer variableThe type()
function helps identify the following built-in types:
- int: Integer type
- float: Floating-point type
- str: String type
- list: List type
- tuple: Tuple type
- dict: Dictionary type
In addition to the above, the type()
function is a clear and helpful tool for beginners learning Python, as well as for debugging code.
Checking Variable Types
In Python, variables don’t require an explicit type declaration. However, there are times when it is necessary to check a variable’s type. This is especially important when working with multiple data types at the same time. The type()
function is ideal for checking the type of variables in such cases.
a = 4096
b = 40.96
c = "4096MB"
d = [1024, 2048, 4096]
print(type(a))
print(type(b))
print(type(c))
print(type(d))
In this example, each variable holds a different type of data. As shown in the figure below, we can use type()
to check the type of each variable.
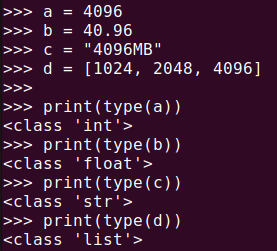
int
, float
, str
, and list
types using Python type()
Using type()
with Conditional Statements
The type()
function becomes especially powerful when used with conditional statements. When you need to perform different actions depending on the type of data, you can efficiently write code using type()
.
data = [4096, "4096MB", 40.96]
for item in data:
if type(item) == int:
print(f"{item} is integer.")
elif type(item) == float:
print(f"{item} is float.")
elif type(item) == str:
print(f"{item} is string.")
This code checks each item in the list and prints a message according to the data type. The type()
function helps determine the type of each data and branches the logic accordingly, as illustrated in the following figure.
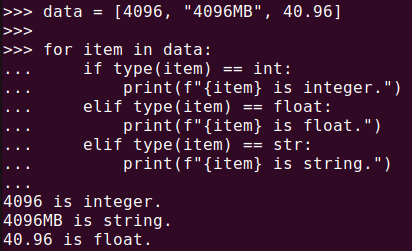
type()
Dynamic Class Creation
Beyond simply returning the type of an object, the type()
function has a special feature: it can dynamically create classes. This capability is useful primarily when you want to create new classes at runtime.
MyClass = type('MyClass', (object,), {'size': 4096})
obj = MyClass()
print(obj.size)
type(obj)
The code above uses type()
to dynamically create a class named MyClass
. You can pass the class name, the parent class to inherit from, and class attributes as arguments to instantly create the class. Additionally, in line 5, the type of the obj
object, which is not a built-in type but a user-defined class MyClass
, is checked.
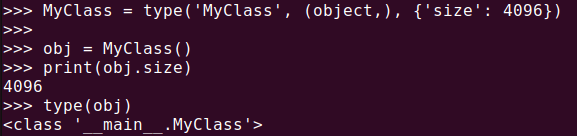
type()
Caution
While the type()
function is very useful, in some cases, using the isinstance()
function for type comparisons is more appropriate. The type()
function checks only the exact type, but isinstance()
also considers inheritance relationships when determining the type.
a = 4096
print(type(a) == int)
print(isinstance(a, int))
class Animal:
pass
class Dog(Animal):
pass
d = Dog()
print(type(d) == Animal)
print(isinstance(d, Animal))
As shown in the figure below, isinstance()
can verify if an object is an instance of a parent class. If you need to consider inheritance relationships when checking types, it’s better to use isinstance()
rather than type()
.
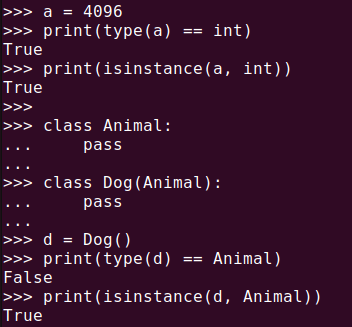
isinstance()
instead of type()
to check for parent class instancesSummary
The type()
function is a powerful tool in Python, useful for checking object types or dynamically creating classes. It is particularly helpful when dealing with multiple data types and when conditional processing based on types is required. However, when inheritance relationships need to be considered, isinstance()
is more appropriate than type()
. Nonetheless, if you need to check for an exact match of a specific class, using type()
provides a clear and precise confirmation.