Python tuples are similar to lists but differ in that they are immutable, meaning their values cannot be changed once created. In this post, we will explore how to use Python’s tuple
in detail, along with different ways to utilize tuples and precautions to consider.
Table of Contents
What is a Python tuple?
A tuple is a data type that allows you to group multiple values together. Unlike a list, tuples are classified as immutable, meaning once created, their values cannot be modified. You cannot change or delete elements from a tuple.
(1, 2, 3)
In the above example, my_tuple
is a tuple containing the values 1, 2, and 3. Tuples are created using parentheses ()
. If you want to create a tuple with only one element, you must add a comma (,) to make Python recognize it as a tuple.
type((5))
type((5,))
Without the comma, Python treats it as a regular integer. As shown in the figure below, adding a comma ensures that it is processed as a tuple, while omitting it treats the value as an integer.
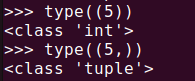
Characteristics of Tuples
Tuples have the following key characteristics:
- Immutability: Once a tuple is created, it cannot be modified. You cannot change, add, or remove elements.
- Ordered: Tuples remember the order in which elements were added. You can easily access elements using their index.
- Duplicates allowed: You can add duplicate values within a tuple.
Difference Between Tuples and Lists
Although tuples and lists are quite similar, the main difference lies in mutability. While lists can have their values changed freely, tuples cannot be modified. This makes tuples useful for storing data that doesn’t need to change, such as coordinates or dates.
Additionally, tuples consume less memory and have faster processing speeds compared to lists. If your program doesn’t require modifying values, using tuples instead of lists can improve performance.
How to Use Tuples
Creating a Tuple
You can create tuples in several ways, but the most common method is by using parentheses ()
.
tuple1 = (1, 2, 3)
tuple2 = ('a', 'b', 'c')
Creating an Empty Tuple
You can create an empty tuple, which contains no elements.
empty_tuple = ()
Alternatively, you can use the tuple() function to create an empty tuple.
empty_tuple = tuple()
Accessing Tuple Elements
You can access tuple elements using an index. Python’s index system starts at 0.
my_tuple = (10, 20, 30)
print("index 0: ", my_tuple[0])
print("index 1: ", my_tuple[1])
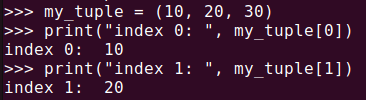
Tuple Unpacking
Unpacking is a method where you assign the elements of a tuple to individual variables. It simplifies the process of extracting values from a tuple.
def get_width_and_height():
width = 250
height = 450
return (width, height)
width, height = get_width_and_height()
print(width)
print(height)
As shown in the image below, you can separate the tuple values and assign them to individual variables, width and height.
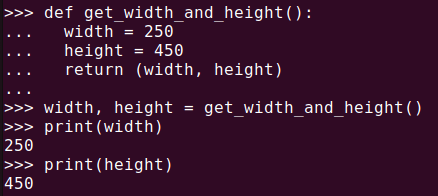
Finding the Length of a Tuple
You can use the len() function to find the length of a tuple, just like with lists.
my_tuple = (1, 3, 7, 4, 2, 3, 4, 5)
print(len(my_tuple))
Combining Tuples
Similar to lists, tuples can be concatenated. The + operator is used to combine two or more tuples. However, unlike lists, tuples are immutable, meaning you cannot modify an existing tuple using methods like extend(). A new tuple is created whenever concatenation occurs.
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
combined_tuple = tuple1 + tuple2
print(combined_tuple)
Like lists, you can use the addition operator (+) to combine two tuples into one.
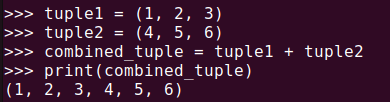
+
operatorSlicing Tuples
Like lists, you can extract parts of a tuple using slicing. This is done by specifying an index range.
my_tuple = (8, 2, 9, 3, 5, 1, 7, 4)
print(my_tuple[3:6])
The elements starting from index 3 (value: 3) up to, but not including, index 6 (value: 7) are returned, resulting in (3, 5, 1).

Practical Uses of Tuples
Tuples are particularly useful when storing fixed data or when a function needs to return multiple values.
- Returning Multiple Values: When a function returns multiple values, it usually returns them as a tuple.
def get_min_max(numbers):
return min(numbers), max(numbers)
result = get_min_max([80, 20, 130, 60])
print(result)
min_value, max_value = get_min_max([80, 20, 130, 60]) # Unpacking tuple
print(min_value, max_value)
As shown in the figure below, the minimum and maximum values are returned as a tuple. We’ve also explored how to unpack these values into the variables min_value and max_value.

Precautions When Using Tuples
Since tuples are immutable, if you need to modify values, it’s better to use lists instead. Also, when creating a tuple with just one element, always remember to include a comma (,). Without the comma, Python will interpret it as a single value, not a tuple.
Summary
Tuples are an immutable data type frequently used in Python when data does not need to be changed. While similar to lists, tuples cannot be modified once created, offering benefits like memory efficiency and faster processing. Their versatility in tasks such as unpacking, slicing, and returning multiple values makes them a valuable tool for writing concise and efficient code. By utilizing tuples effectively, you can improve both the performance and readability of your programs.