The Python str()
function is one of the most fundamental functions for handling strings. It converts various data types into strings and is frequently used in programming. In this post, we will explore the basic usage and applications of the str()
function.
Table of Contents
What is the str() Function?
The str()
function is a built-in Python function that converts various data types, such as integers, floats, lists, and tuples, into strings. A string is a sequence of characters, enclosed in single ('
) or double ("
) quotes in Python.
For example, using the str()
function to convert the numeric data 123
into a string results in '123'
. It is important to note the difference between numbers and strings—numbers can be used for calculations, while strings are treated as text.
num = 123
str_num = str(num)
print(str_num, type(str_num))
In the above code, the type()
function shows the data type. num
is an integer (int
), but after using str()
, str_num
is now a string (str
).
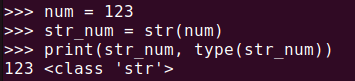
Various Uses of the str() Function
Converting Numbers to Strings
The most basic use of the str()
function is converting numbers into strings. For instance, you can convert numbers to strings when printing calculated values, allowing them to be displayed with other strings.
num1 = 5
num2 = 8
result = str(num1) + " and " + str(num2) + " sum to " + str(num1 + num2)
print(result)
In this code, num1
and num2
are converted to strings and displayed with other text. This makes it possible to output both numbers and strings together.

Converting Lists and Tuples to Strings
Data types like lists and tuples can also be easily converted to strings using str()
. This is particularly useful when storing or printing data.
my_list = [1, 2, 3]
my_tuple = (4, 5, 6)
print("List: " + str(my_list))
print("Tuple: " + str(my_tuple))
Using str()
, lists or tuples are converted into strings where each element remains as part of the list or tuple structure.
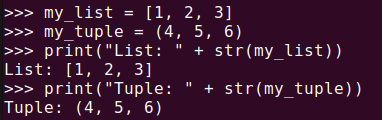
Converting Boolean Values to Strings
A boolean value represents either True
or False
. The str()
function can convert boolean values to strings, which is particularly useful when displaying results of conditions to users.
is_active = True
print("Active status: " + str(is_active))
In the above example, the True
value is converted into the string "True"
and then displayed.
Cautions When Using str()
Distinguishing Between Numeric Operations and String Concatenation
A key caution when mixing numbers and strings is that while numbers can be used for calculations, strings cannot. For example, if you want to concatenate a number with a string using the +
operator, you must first convert the number using str()
.
If you attempt to concatenate a number with a string using the +
operator without converting the number to a string, a TypeError
will occur. The following code illustrates that numeric and string concatenation is not allowed.
Python3 + " eggs"
You will encounter a TypeError: unsupported operand type(s) for +: 'int' and 'str'
error, as shown in the figure below.

Now, consider the following code where a string precedes the number.
"images of " + 3
This will result in the error message 'TypeError: can only concatenate str (not "int") to str'
.

Therefore, when concatenating a number with a string using the +
operator, you must convert the number to a string using str()
.
Conversion Differences Depending on Data Types
Although all data types can be converted using str()
, the results may vary depending on the data type. For instance, complex data types like lists or dictionaries are converted into strings while retaining their structure, so readability should be taken into consideration.
my_dict = {"name": "John", "age": 25}
print(str(my_dict))
Additionally, when converting boolean values like True
or False
into strings and then converting them back using bool()
, both will be treated as True
. This behavior requires careful attention.
Applications of the str() Function
Usage in File I/O
The str()
function is also useful when storing data in files. Since files are usually saved as text, numbers or lists need to be converted into strings before they can be stored.
my_data = [5, 10, 23, 18, 38, 7]
with open('data.txt', 'w') as file:
file.write(str(my_data))
In the code above, the list my_data
is converted into a string before being saved to a file, as shown in the figure below.

Usage in Web Development
In web development, the python str()
function is often used. For example, when sending user-input data to a server, it is common to convert various data types into strings. This is especially true for JSON data, where types must be converted into strings for transmission.
Summary
The Python str() function is a simple yet powerful tool for converting various data types into strings. You can easily print or save different types of data such as numbers, lists, or booleans by converting them into strings, allowing for more concise code. Additionally, being cautious of type conversions can prevent errors during operations. Given its frequent use in file I/O and web development, the str()
function is a fundamental tool that you should definitely master.