Python setattr() function is particularly useful when you need to dynamically change the attributes of an object. This function takes the attribute name as a string and sets the attribute value accordingly. In this post, we’ll go over the basic usage of the setattr()
function, along with various practical applications.
Table of Contents
What is Python setattr()
Function?
The setattr()
function is one of Python’s built-in functions that allows you to dynamically set an object’s attribute. In other words, you can change an object’s attribute value or even add new attributes during the execution of a program. For example, it’s highly effective when you need to modify an instance attribute of a defined class or dynamically add new attributes.
Basic Syntax
The basic syntax of setattr()
is as follows:
setattr(object, name, value)
object
: The object whose attribute you want to changename
: The name of the attribute you want to set (provided as a string)value
: The value you want to set
Let’s look at a simple example where we use setattr()
to modify the attributes of an object:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("Emma", 25)
setattr(person, 'age', 30)
print(person.age)
setattr(person, 'gender', 'Female')
print(person.gender)
In the above code, setattr()
is used to modify the existing age
attribute to 30, and a new gender
attribute is added. setattr()
is particularly useful for dynamically manipulating attributes in this way.
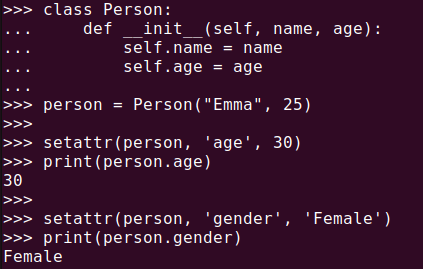
setattr()
to change attribute values and add new attributesHandling Multiple Attributes at Once
It’s especially effective when there is a possibility of changing attribute names or when you need to handle multiple attributes simultaneously. The following example shows how you can use a loop to set multiple attributes on an object at once.
class Product:
pass
product = Product()
attributes = {'name': 'T-shirt', 'price': 37, 'brand': 'BrandName'}
for key, value in attributes.items():
setattr(product, key, value)
print(product.name)
print(product.price)
print(product.brand)
As seen above, managing multiple attributes in a dictionary form allows you to set them all at once. This is far more efficient and easier to maintain compared to setting each attribute individually.
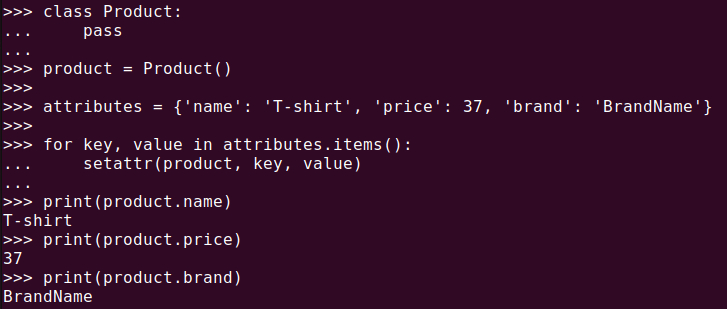
setattr()
to dynamically set attributes with dictionary keys and valuesTip: Using getattr()
Alongside setattr()
A function that is often used in conjunction with setattr()
is getattr()
. This function retrieves the value of an attribute from an object. When combined, these two functions can provide a powerful way to dynamically manipulate an object’s attributes.
class Car:
def __init__(self, model, year):
self.model = model
self.year = year
car = Car("Corolla", 2020)
setattr(car, 'color', 'Red')
color = getattr(car, 'color', 'Unknown')
print(color)
As shown above, by using getattr()
with setattr()
, you can safely write code without worrying about whether the attribute exists or not.
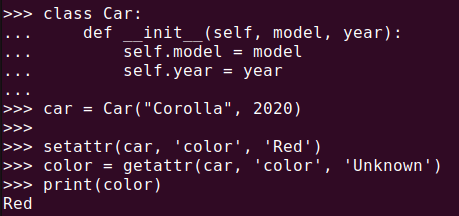
setattr()
with getattr()
Key Considerations
- Be Cautious with Attribute Names: Since
setattr()
accepts attribute names as strings, providing an incorrect string could lead to unintended behavior. For instance, if you pass the name of an attribute that doesn’t exist in the class, that attribute will be newly added. While this can be helpful at times, it may cause bugs if used incorrectly, so it’s important to be careful. - Using with Inheritance: You can also use
setattr()
to modify parent class attributes in inherited classes. However, if the inheritance structure is complex, altering attributes may cause unexpected issues, so caution is advised.
Summary
Python setattr()
function is a highly useful tool when you need to dynamically set object attributes or add new ones. It increases the flexibility of your code and reduces repetitive tasks. It’s especially advantageous when handling dynamic attributes or managing multiple attributes at once.
However, overusing it can make the structure of your objects more complex, so it should be used with care. By properly leveraging setattr()
alongside getattr()
, you can handle object attributes safely and efficiently.