The Python set() built-in function is used to create sets, allowing for the storage of data without duplicates and easy handling of various operations. Python offers a variety of data structures, and among them, a set represents a collection that is quite similar to the concept of sets in mathematics. In this post, we will explore how to use set() in detail.
Table of Contents
Characteristics of set()
The set data type has the following key characteristics:
- No duplicates allowed: If there are multiple identical values, only one will be stored. For example, when you convert
[1, 2, 2, 3]
into a set, you get{1, 2, 3}
. - Unordered: Unlike lists, the elements are not stored in a particular order, so indexing and slicing are not possible.
- Mutable: New values can be added or existing values can be removed from a set.
These features make set a useful tool for storing unique values, performing fast searches, and eliminating duplicates.
How to Create a set
There are two main ways to create a set in Python. One method is to use the set() function, and the other is to use curly braces {}
.
Pythonset([1, 2, 3, 4, 5])
# or
{1, 2, 3, 4, 5}
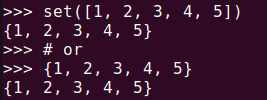
However, when creating an empty set, you must use the set() function. Using curly braces {}
will create a dictionary instead of a set.
type(set())
type({})
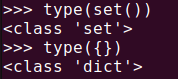
How to Use set
set is mainly used to handle operations such as removing duplicates, finding intersections, and performing unions. Each operation can be performed easily with methods or operators in Python.
Removing Duplicates
You can use a set to remove duplicate values from a list or any other sequence.
my_list = [1, 2, 2, 3, 2, 3, 5, 4, 4, 5]
my_set = set(my_list)
print(my_set)
As shown in the following figure, a set allows you to easily remove duplicate elements from a list.

Union
Union combines two or more sets into one. You can use the |
operator or the union() method to perform a union.
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result = set1 | set2
print(result)
result = set1.union(set2)
print(result)
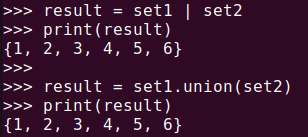
Intersection
Intersection finds the common elements between two sets. You can use the &
operator or the intersection() method.
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result = set1 & set2
print(result)
result = set1.intersection(set2)
print(result)
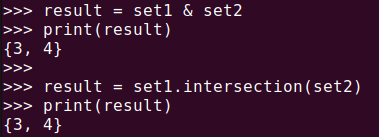
Difference
The difference finds the elements in one set that are not present in another. You can use the -
operator or the difference() method.
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result = set1 - set2
print(result)
result = set1.difference(set2)
print(result)
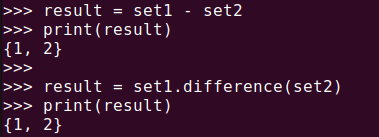
Symmetric Difference
The symmetric difference finds the elements that are in either of the sets but not in both. You can use the ^
operator or the symmetric_difference() method.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
result = set1 ^ set2
print(result)
result = set1.symmetric_difference(set2)
print(result)
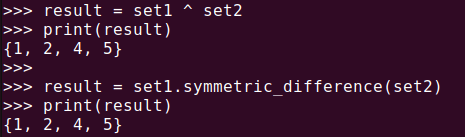
Precautions
Since set is an unordered data type, you cannot use methods such as indexing or slicing, which are common in lists. For example, trying to access the first element with my_set[0]
will result in an error. To iterate over elements in a set, you need to use a for loop.
my_set = {1, 2, 3}
for element in my_set:
print(element)
Additionally, the elements of a set must be immutable (unchangeable) data types. Therefore, mutable objects like lists or dictionaries cannot be used as elements of a set.
Summary
Python’s set() function is a useful tool for managing duplicate data and quickly performing set operations. It supports various operations such as union, intersection, and difference, and is particularly powerful when it comes to eliminating duplicates or finding unique values. Practice using set to write more efficient code.