The Python ord()
function is a very useful tool that returns the Unicode value of a given character. In this post, we’ll explore the basic usage of the ord()
function, along with some practical applications that can be useful in real-life scenarios. We’ll also cover potential pitfalls and hidden features in each case.
Table of Contents
What is the Python ord() Function?
Python ord()
function provides a simple yet powerful functionality. It takes a single character as input and returns its Unicode value. Unicode is a universal character encoding standard that assigns a unique numeric value to each character across all written languages. For example, the Unicode value of the lowercase English letter ‘a’ is 97, and the Unicode value of ‘A’ is 65.
How to Use ord()
The usage of the ord()
function is quite simple. You provide a single character as input, and the function will return its corresponding Unicode value. Here’s a basic example:
ord('a')
ord('A')
ord('あ')
ord('爱')
ord('가')
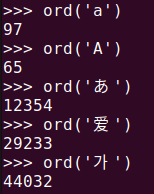
As shown, ord()
instantly returns the Unicode value for a single character. However, be careful—ord()
can only process single characters. If you pass an entire string, it will raise an error.
ord('ab')
When a string with more than one character is used, the error message “TypeError: ord() expected a character, but string of length 2 found” will inform you that only a single character should be used.

The Difference Between Unicode and ASCII
The value returned by the ord()
function is a Unicode value. It’s essential to understand the difference between Unicode and ASCII.
- ASCII (American Standard Code for Information Interchange): This is a character encoding standard for representing text in computers, limited to 128 characters, including English letters, numbers, and symbols. It is insufficient for representing all languages worldwide.
- Unicode: A more comprehensive encoding standard that represents characters from virtually every language, including Korean, Chinese, Arabic, and many others.
Thus, the ord()
function isn’t restricted to ASCII characters—it can convert characters from a wide range of languages into their Unicode equivalents.
Practical Applications of the ord() Function
Finding Alphabet Position
The ord()
function is very useful when you need to find the position of an alphabet letter. For instance, ‘a’ is the first letter, and ‘b’ is the second letter of the alphabet. Using ord()
, we can easily determine the letter’s position.
def alphabet_position(letter):
return ord(letter.lower()) - ord('a') + 1
print(alphabet_position('A'))
print(alphabet_position('B'))
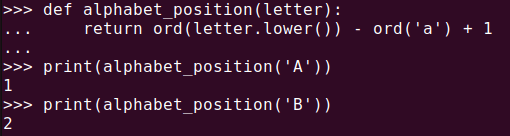
Calculating Distance Between Characters
You can also calculate the “distance” between two characters by measuring the difference in their Unicode values. For example, there are two positions between ‘a’ and ‘c’.
def char_distance(char1, char2):
return abs(ord(char1) - ord(char2))
print(char_distance('a', 'c'))
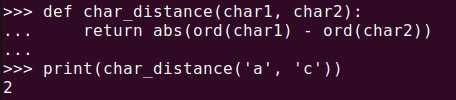
This method can be used in encryption algorithms, string comparisons, and various other applications.
Strengthening Password Security
You can analyze the complexity of a user’s password by evaluating the range of Unicode values used. For example, calculating the sum of the Unicode values of characters in a password can help gauge its strength.
def password_strength(password):
strength = 0
for char in password:
strength += ord(char)
return strength
password_strength("abc123")
password_strength("aBc123$")
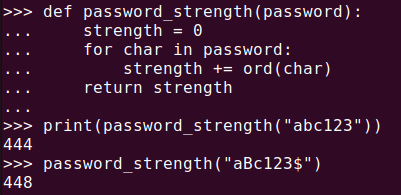
In this way, the ord()
function can be utilized to analyze password diversity and act as a metric for strengthening security.
Things to Be Aware Of
- The
ord()
function can only process single characters. If you input a string, an error will occur, so you need to handle strings by separating them into individual characters. - The value returned is always an integer, so it’s not applicable for floating-point operations.
Summary
Python’s ord()
function offers a simple yet powerful way to work with characters by returning their corresponding Unicode values. Whether you’re finding the position of a letter, calculating the distance between characters, or evaluating password strength, the ord()
function can be quite useful in a variety of applications. However, keep in mind that it only works with single characters.
With such a versatile function, there are many ways to use it in Python for handling strings. Consider exploring ord()
in your next project involving text processing or security.