In this post, we will explain how to use Python oct()
built-in function and explore how it can be applied in real-world scenarios. Python is a high-level programming language that allows for easy handling of various mathematical operations. Among these, handling different numeral systems (bases) is crucial, and we will focus on the oct()
function used for this purpose. Additionally, we will provide explanations of relevant terms for beginner developers, along with useful ways to utilize this function.
Table of Contents
What is the Python oct() Function?
The oct()
function is a built-in Python function that converts numbers into octal (base-8) format. In Python, integers are represented in decimal (base-10) by default. However, using the oct()
function, you can convert a number into its octal representation, and the result is returned as a string. Octal uses numbers from 0 to 7 and is commonly used in system programming and memory address handling.
Example
num = 10
print(oct(num))
When this code is executed, the result '0o12'
will be printed, indicating that the decimal number 10 has been converted to the octal equivalent of 12. The prefix 0o
denotes that the number is in octal format.
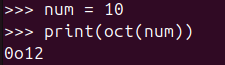
What is Octal?
Octal (base-8) is a numeral system that uses digits from 0 to 7. For example, in decimal, the number 8 is represented as 10
in octal, and 10 in decimal is 12
in octal. Although binary (base-2) is widely used for storing or transmitting data, octal and hexadecimal (base-16) are often used to improve readability.
How to Use the oct() Function
Basic Usage
The oct()
function simply takes an integer and converts it to an octal string. The syntax is as follows:
oct(integer)
Here, the integer
represents the number to be converted. Note that if you pass a non-integer value (e.g., a float), a TypeError
will be raised, so you need to be cautious.
Handling Negative Numbers
The oct()
function can handle negative numbers as well. When a negative number is passed, it returns the octal representation prefixed with a negative sign.
print(oct(-8))
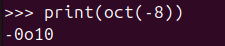
Applications of the oct() Function
Usage in System Programming
In system programming, octal numbers are often used to represent memory addresses or file permissions. Particularly in Linux and Unix-based systems, file permissions are set using octal values. The oct()
function is very useful in these cases.
File Permission Example
In Linux, file permissions can be set using the chmod
command along with octal values. For example, chmod 755
grants read, write, and execute permissions to the file owner, and read and execute permissions to the group and others. When working with file permissions in Python, the oct()
function can be used to easily verify the permission values.
file_mode = 0o755
print(oct(file_mode))
The output is as follows.
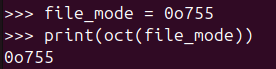
Usage in Network Programming
In network programming, when dealing with IP addresses or port numbers, you may need to convert between numeral systems. The oct()
function can simplify the conversion of IP addresses or port numbers to octal format for processing.
Conversion Between Number Systems
There are times when conversions between different numeral systems are necessary. For example, you might need to convert a decimal number to octal and then to hexadecimal. By using the oct()
function alongside other conversion functions, you can handle this efficiently.
num = 15
octal_value = oct(num)
hex_value = hex(int(octal_value, 8))
print(f"Decimal: {num}, Octal: {octal_value}, Hexadecimal: {hex_value}")
This code converts the decimal number 15 to octal and hexadecimal, displaying the results.

Important Considerations When Using the oct() Function
Note on String Output
The oct()
function returns the converted value as a string. If you need to perform numeric operations on it, you must convert it back to an integer. You can use the int()
function to convert the octal string back into a decimal integer.
octal_value = oct(8)
print(octal_value)
int_value = int(octal_value, 8)
print(int_value)
The output is as follows.
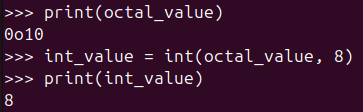
Handling Floats and Other Data Types
The oct()
function only works with integers. If you pass a float or any non-integer value, it will raise a TypeError
. Always check the data type before using the function.
print(oct(3.14)) # Raises a TypeError
If you pass a floating-point number, Python will display an error message such as “TypeError: ‘float’ object cannot be interpreted as an integer,” as shown below.

Summary
The oct()
function in Python provides a simple but highly useful way to convert numbers into octal format. It’s frequently used in system programming, file permission settings, and network programming. The function returns the result as a string, and when used in combination with other numeral system conversion functions, it becomes an even more powerful tool. When using the oct()
function, be mindful of the data type to prevent errors, and ensure that the number you’re converting is in the correct format.