The Python list()
function is one of the most powerful data structures available. This post introduces the basic usage of list()
along with efficient methods of utilizing it. Whether you’re a beginner or an experienced programmer, I’ll explain essential concepts in an easy-to-understand way, so keep reading until the end.
Table of Contents
What is list()?
The list()
function in Python is used to create a list data type. A list is a mutable sequence data structure that can store multiple values in a single variable. “Mutable” means that you can add or remove values from the list. While similar to arrays, Python lists can store various types of data. For instance, you can store integers, strings, and floats in a single list.
Basic Usage of list()
There are two main ways to create a list:
- Using square brackets:
my_list = [1, 2, 3, 4]
- Using the
list()
function:my_list = list([1, 2, 3, 4])
Both methods create the same list. The list()
function is also used to create an empty list or to convert other iterable objects into a list.
# Creating an empty list
empty_list = []
print(empty_list)
empty_list = list()
print(empty_list)
# Converting a string to a list
str_list = list("hello")
print(str_list)
In the figure below, we can see the result of creating an empty list and converting a string into a list.
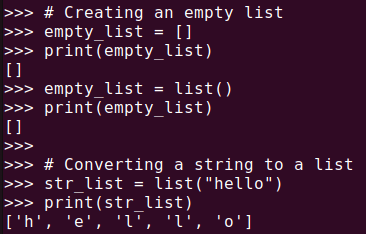
Key Features of Lists
Adding Elements: append()
To add new elements to a list, use the append()
method, which adds an element to the end of the list.
my_list = [1, 2, 3]
my_list.append(4)
print(my_list)
In the figure below, you can see that the value 4 has been added to the end of my_list
.
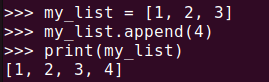
Adding Multiple Elements: extend()
The extend()
method allows you to add multiple elements from another list or iterable to the list at once.
my_list.extend([5, 6])
print(my_list)
In the figure below, you can see that the values of the list [5, 6]
have been added to the end of my_list
.
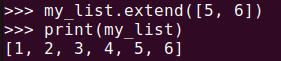
Inserting an Element: insert()
To insert an element at a specific position, use the insert()
method. You can specify the index where you want to insert the value.
my_list = [1, 2, 4]
my_list.insert(2, 3) # Insert number 3 at index 2
print(my_list)
In the figure below, you can see that the number 3 was inserted into index 2, where the value 4 used to be, and the value 4 was pushed to index 3.

Removing Elements: remove(), pop(), del
There are several ways to remove elements from a list. You can use the remove()
or pop()
methods, or you can use the del
command in Python.
remove()
: Removes the first occurrence of the specified element from the list.pop()
: Removes and returns the element at the specified index. If no index is specified, the last element is removed.del
: Removes the element at the specified index using thedel
command.
Pythonmy_list = [1, 2, 3, 4, 5, 2, 3, 4]
my_list.remove(3)
print(my_list)
popped_value = my_list.pop(1)
print(my_list)
print(popped_value)
popped_value = my_list.pop()
print(my_list)
print(popped_value)
del my_list[0]
print(my_list)
Lines 1-3 use the remove()
method to delete the first occurrence of the number 3. Lines 5-7 use the pop()
method to remove the value at index 1. Lines 8-9 use the pop()
method to remove the last element. Lines 12-13 use the del
command to remove the value at index 0. Below is the result of executing the above code.
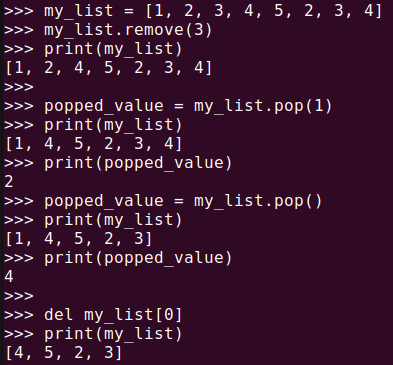
Sorting a List: sort(), sorted()
To sort a list in ascending order, use the sort()
method. If you want to sort it in descending order, add the reverse=True
option.
my_list = [3, 1, 5, 4, 2]
my_list.sort()
print(my_list)
my_list.sort(reverse=True)
print(my_list)
In the figure below, you can see the results of sorting my_list
in ascending order using the sort()
method, and sorting it in descending order by applying reverse=True
to the sort()
method.
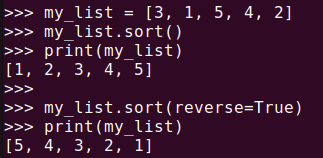
The sorted()
function returns a new sorted list, while the sort()
method modifies the original list.
my_list = [3, 1, 5, 4, 2]
sorted_list = sorted(my_list)
print(my_list)
print(sorted_list)
In the figure below, my_list
remains unchanged, and only sorted_list
contains the sorted values.
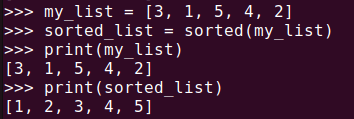
Reversing a List: reverse()
The reverse()
method reverses the order of elements in the list. It’s important to note that this method does not sort the list but simply reverses its current order.
my_list = [1, 3, 5, 2, 4]
my_list.reverse()
print(my_list)
In the following figure, you can see that the first line of the list has been reversed without being sorted.
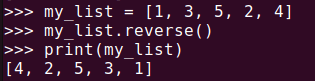
Getting the Length of a List: len()
To find the length of a list, or the number of elements in it, use the len()
function.
my_list = [1, 2, 3, 4, 3, 2, 5]
print(len(my_list))
In the next figure, you can see that the length of the list is 7.

Checking for Membership: in Operator
To check if a list contains a specific value, use the in
operator.
my_list = [1, 2, 3, 4]
print(3 in my_list)
print(5 in my_list)
In the figure below, you can see that 3 is an element of my_list
, so True
is returned, while 5 is not an element of my_list
, so False
is returned.
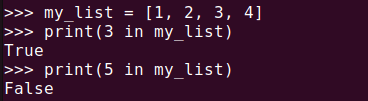
Copying a List
You should not use the assignment operator to copy a list, as it will make both variables point to the same object. Instead, use the copy()
method or the list()
function for a shallow copy, or use deepcopy()
for a deep copy. Below is an example of shallow copying:
original = [1, 2, 3]
copy_list = original.copy()
print(copy_list)
In the figure below, you can see an example of copying a list using shallow copy.
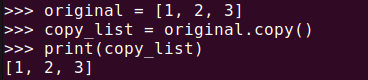
Precautions
- Since lists are mutable, methods that modify the list will change it directly. If you need to preserve the original data, it’s best to make a copy of the list before performing any modifications.
- Lists start their indexing at 0, so always keep this in mind when working with list indices.
Summary
Python’s list()
function is an incredibly useful tool for storing and managing data. With methods like append()
, insert()
, and remove()
, you can manipulate lists flexibly, and with sort()
and reverse()
, you can easily adjust the order of elements. Lists are one of the most widely used data types in Python, so mastering the functions and methods introduced in this guide will significantly improve your coding and problem-solving skills.