The Python issubclass()
function is used to check whether a specific class is a subclass of another. In Python, object-oriented programming (OOP) allows you to write structured code using classes and objects. Before diving into the usage of the issubclass()
function, let’s first go over the basic concepts of classes and subclasses.
Table of Contents
What Are Classes and Subclasses?
A class can be seen as a blueprint for creating objects. In Python, you can extend or modify an existing class through inheritance, allowing you to create child classes or subclasses. A class that inherits is called a subclass, while the class being inherited from is known as the superclass or parent class.
For instance, when there’s a parent class called Animal
, a child class Cat
can inherit from it and become a subclass.
class Animal:
pass
class Cat(Animal):
pass
In this code, Cat
is a subclass of Animal
.
What Is the Python issubclass() Function?
issubclass()
is a built-in function that takes two arguments: the first is the class you want to check (the subclass), and the second is the superclass. If the first class is a subclass of the second, it returns True
; otherwise, it returns False
. Here’s the basic syntax:
issubclass(class_to_check, base_class)
Let’s take a look at an example where Animal
is the parent class, and Cat
is a child class that inherits from Animal
. We’ll check if Cat
is a subclass of Animal
, but not vice versa.
class Animal:
pass
class Cat(Animal):
pass
print(issubclass(Cat, Animal))
print(issubclass(Animal, Cat))
Since Cat
is a subclass of Animal
, the first call returns True
. On the other hand, Animal
is not a subclass of Cat
, so the second call returns False
.
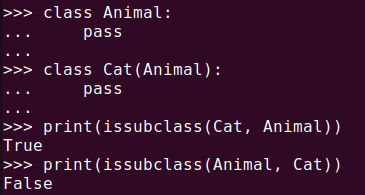
Checking Multiple Classes Using Tuples
The issubclass()
function can also accept a tuple of classes as the second argument. In this case, if the first class is a subclass of any of the classes in the tuple, it returns True
.
class Animal:
pass
class Dog(Animal):
pass
class Cat(Animal):
pass
print(issubclass(Dog, (Animal, Cat)))
print(issubclass(Cat, (Dog, int)))
In the example below, since Dog
is a subclass of Animal
, the first call returns True
. However, Cat
is neither a subclass of Dog
nor int
, so the second call returns False
.
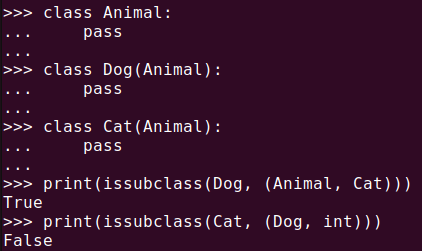
issubclass()
Multiple Inheritance
Python supports multiple inheritance, meaning a class can inherit from more than one superclass. Even in such cases, the issubclass()
function works correctly.
class Animal:
pass
class Mammal:
pass
class Cat(Animal, Mammal):
pass
print(issubclass(Cat, Animal))
print(issubclass(Cat, Mammal))
In this example, since Cat
inherits from both Animal
and Mammal
, both checks return True
.
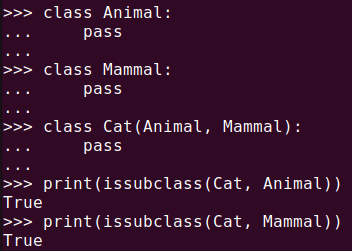
issubclass()
correctly handles multiple inheritance in PythonCaution: The First Argument Must Be a Class
The first argument to issubclass()
must be a class. If you pass an object instead of a class, a TypeError
will occur.
class Animal:
pass
a = Animal()
print(issubclass(a, Animal))
In this case, a
is not a class but an instance of Animal
, so Python raises a “TypeError: issubclass() arg 1 must be a class
“, clearly stating that the first argument must be a class.
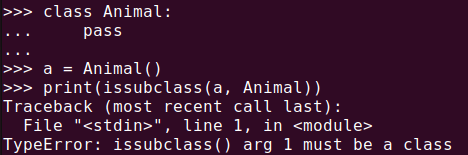
TypeError
occurs if the first argument to issubclass()
is an objectUseful Tips for Using issubclass()
- Check Class Hierarchies:
issubclass()
is helpful for understanding class inheritance relationships, especially in large projects with multiple classes. - Unit Testing: You can use
issubclass()
in unit tests to ensure that classes are inheriting as expected. If the inheritance hierarchy is incorrect, this could lead to bugs, so verifying it in advance is useful. - Polymorphism: When implementing polymorphism, you can use
issubclass()
to check if a class inherits from a parent class or a specific interface. This helps prevent errors when dealing with functions that handle different types of objects.
Summary
The issubclass()
function is a powerful tool for verifying inheritance relationships between classes in Python. By using it, you can clarify class structures and easily validate inheritance in your code. This function is especially useful when dealing with complex class hierarchies or multiple inheritance scenarios. Additionally, it plays an important role in unit testing and polymorphism.
Remember to always use classes as the arguments for issubclass()
. Passing objects instead of classes will result in a TypeError
. By mastering issubclass()
, you can implement object-oriented programming in Python more effectively.