The Python isinstance()
function in Python is used to determine the type of an object, similar to the type()
function. The isinstance()
function checks whether a specific object is an instance of a given class or data type. This can help improve code reliability and simplify branching based on type. In this post, we’ll explore how to use the isinstance()
function and its practical applications.
Table of Contents
Basic Usage of Python isinstance()
The isinstance()
function takes two arguments: the first is the object to be checked, and the second is the class or data type to be compared. The function returns True
if the object is an instance of the specified class, and False
otherwise. Here’s the basic usage:
isinstance(object, class)
In the example below, we check if the variable x
is of type int
.
x = 4096
print(isinstance(x, int))
Since the variable x
is an integer (int
), the output will be True
.

Checking Multiple Classes
The second argument of isinstance()
can be a single class or a tuple of multiple classes. In this case, the function returns True
if the object is an instance of any of the classes in the tuple.
x = 40.96
y = 4096
print(isinstance(x, (int, float)))
print(isinstance(y, (int, float)))
In line 3, since x
is a float
(with the value 40.96
), the function returns True
. In line 4, since y
is an int
(with the value 4096
), it also returns True
.
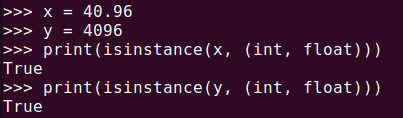
The advantage of checking multiple classes is that it is useful when a function or method needs to handle various data types. This can make the code more flexible and reduce the chances of processing incorrect types of data.
Why Use isinstance(): Comparison with type()
Python also provides the type()
function to check the type of an object. However, unlike type()
, the isinstance()
function considers inheritance relationships. For example, you can use isinstance()
to check if a subclass inherits from a superclass, but type()
only checks for an exact match.
class Animal:
pass
class Cat(Animal):
pass
cat = Cat()
print(isinstance(cat, Animal))
print(type(cat) == Animal)
The isinstance()
function returns True
because the cat
object is an instance of the Animal
class (inherited by Cat
). However, type()
returns False
because cat
is specifically an instance of the Cat
class.
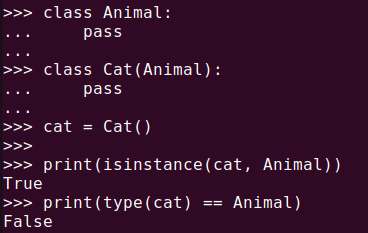
Precautions When Using isinstance()
- Avoid Over-reliance on Type Checking: Python is a dynamically typed language, meaning types do not need to be explicitly declared. Overly strict type checking can reduce Python’s flexibility. Therefore, use
isinstance()
only when absolutely necessary. - Be Careful with Tuple of Classes: When passing a tuple of classes as the second argument, ensure the tuple is correctly formatted. If a list or another data type is passed instead of a tuple, a
TypeError: isinstance() arg 2 must be a type, a tuple of types, or a union
will occur.

Summary
The isinstance()
function is a powerful tool in Python for checking the type of an object. It is frequently used over the type()
function because it works correctly with inheritance relationships. By utilizing isinstance()
, you can enhance code readability and develop functions that handle various data types effectively. However, be mindful not to overuse type checking, as it can limit the dynamic nature of Python.
Use isinstance()
wisely to ensure safe type checking and write more flexible code!