The Python hasattr()
function checks whether a particular object has a specified attribute. Python is a flexible programming language that follows an object-oriented approach. In object-oriented programming, dealing with object attributes is essential. In this post, we will explore how to use the hasattr()
function and explain how it can assist in Python programming through various use cases.
Table of Contents
What is the Python hasattr() function?
The hasattr()
function is a built-in Python function used to check if an object has a specific attribute. This function takes two arguments:
- First argument: The object whose attributes you want to check.
- Second argument: The name of the attribute to check (in string format).
This function returns True
if the attribute exists; otherwise, it returns False
. It is useful for dynamically checking the presence of attributes in objects during runtime.
hasattr(object, 'attribute_name')
Below is an example of the basic usage of the hasattr()
function.
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
my_car = Car('Toyota', 'Corolla')
print(hasattr(my_car, 'brand'))
print(hasattr(my_car, 'color'))
In this code, we define a class called Car
and check whether the object my_car
has the attributes brand
and model
. Since the brand
attribute exists, True
is returned, but since color
does not exist, False
is returned.
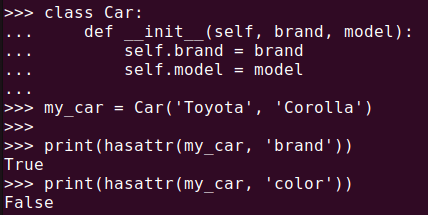
How to Use hasattr()
The hasattr()
function is mainly used when you need to check an object’s dynamic attributes. It allows you to assess an object’s state or attributes during runtime, offering flexibility in handling different situations. Below are some useful scenarios where hasattr()
can be applied.
Dynamic Attribute Assignment
When you don’t know in advance whether an object has a specific attribute, you can use hasattr()
to check the attribute’s existence before dynamically adding it.
class User:
def __init__(self, name):
self.name = name
user = User('Alice')
if not hasattr(user, 'age'):
user.age = 25
print(user.age)
In this code, if the User
object does not have the age
attribute, it dynamically adds it. This allows you to change your program’s logic based on the presence or absence of attributes.
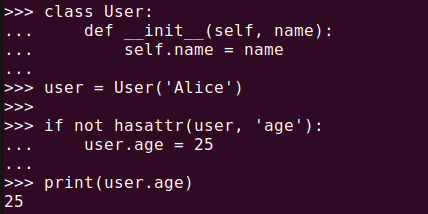
Flexible Handling Based on Attributes
The hasattr()
function is also useful when you need to perform different tasks depending on whether an object has a certain attribute. This is especially helpful in scenarios where different logic must be executed based on the presence of attributes.
class Animal:
def __init__(self, species):
self.species = species
dog = Animal('dog')
if hasattr(dog, 'species'):
print(f"This is a {dog.species}.")
else:
print("Unknown species.")
In this example, we check whether the species
attribute exists, and the output changes accordingly based on the result.
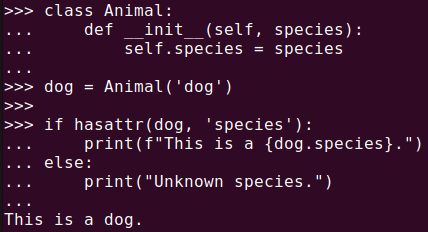
Precautions
While the hasattr()
function is very useful, there are some precautions you should keep in mind when using it.
AttributeError for Non-Existent Attributes
The hasattr()
function checks for the existence of attributes, but just because an attribute does not exist does not mean it is always safe. Attempting to access a non-existent attribute will raise an AttributeError
. Therefore, it is recommended to check for the attribute’s existence with hasattr()
before trying to access it.
class Animal:
def __init__(self, species):
self.species = species
dog = Animal('dog')
if hasattr(dog, 'name'):
print(dog.attribute)
else:
print('Attribute "name" not found')
This code demonstrates how to safely access an attribute. It ensures that if the attribute is missing, an appropriate error is avoided and handled.
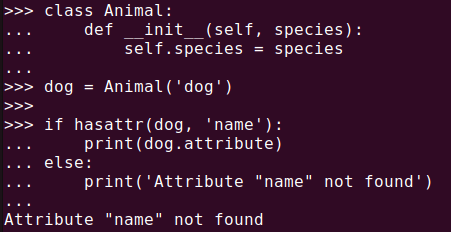
Attribute Names Must Be Strings
The second argument of the hasattr()
function should always be a string representing the attribute’s name. If you pass a non-string value, a TypeError
may be raised. Therefore, make sure to always specify attribute names as strings.
hasattr(dog, dog.name)
hasattr(dog, 'name')
In the incorrect example, attempting to access dog.name
directly without checking if the attribute exists first will raise an AttributeError: 'Animal' object has no attribute 'name'
, indicating that the name
attribute does not exist in the Animal
object.
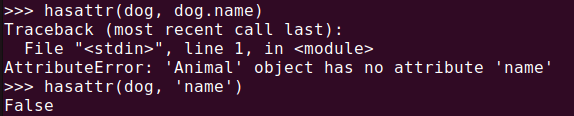
Useful Example: Method Check
The hasattr()
function can check not only for attributes but also for the presence of methods. This allows you to verify if an object implements a particular method.
class Dog:
def bark(self):
print("Woof!")
dog = Dog()
if hasattr(dog, 'bark'):
dog.bark()
In this code, we check whether the object has a bark
method and call it if it exists. This allows for dynamic checking and execution of methods.
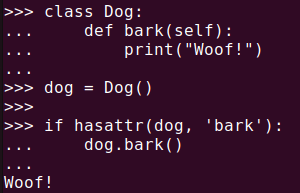
Summary
The Python hasattr()
function is a highly useful tool for checking the existence of attributes in objects. It can be applied in various situations such as dynamic attribute assignment, flexible handling based on attribute existence, and method verification. When using hasattr()
, ensure that attribute names are passed as strings and handle missing attributes appropriately to avoid errors.
By using this function wisely, you can write better object-oriented code and handle object states flexibly in different scenarios.