The Python getattr()
function is a highly useful tool that allows you to dynamically access an object’s member variables or methods. Unlike static coding, it offers a more flexible way to access the attributes of an object, making it applicable in various situations. In this post, we will explore how to use the getattr()
function, its beneficial applications, and precautions to consider when using it.
Table of Contents
What is the Python getattr() Function?
The getattr()
function is primarily used to access specific attributes of an object. Normally, we use the dot operator (.
) to access an object’s attributes, but with getattr()
, you can pass the attribute’s name as a string and access it dynamically. This means you can decide which attribute to access at runtime without hardcoding it beforehand.
Basic Syntax of the getattr() Function
getattr(object, attribute_name, default_value=None)
object
: The object from which you want to retrieve an attribute or method.attribute_name
: The name of the attribute or method you want to access, given as a string.default_value
: The default value to return if the attribute does not exist. If omitted and the attribute doesn’t exist, anAttributeError
will be raised.
Let’s take a look at an example:
class Example:
def __init__(self):
self.name = 'Kyle'
self.age = 23
example = Example()
getattr(example, 'name')
getattr(example, 'age')
getattr(example, 'gender', 'unknown')
In the above example, the getattr()
function successfully accesses the name
and age
attributes of the example
object. For the nonexistent gender
attribute, it returns the default value ‘unknown’.
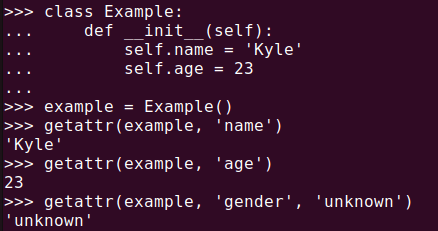
Useful Ways to Dynamically Access Attributes
Dynamic Attribute Access
The getattr()
function becomes incredibly useful when your code needs to behave differently based on user input.
class User:
def __init__(self, name, email):
self.name = name
self.email = email
user = User("John", "john@example.com")
attribute = input("Which attribute would you like to access? (name/email): ")
getattr(user, attribute, "Attribute not found.")
Based on the user’s input, you can dynamically access either the name
or email
attributes.
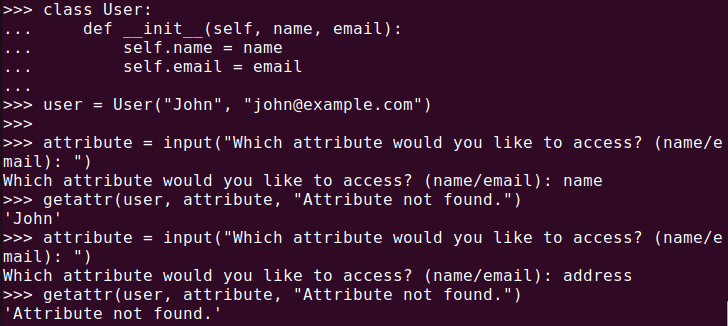
Error Prevention with Default Values
By default, attempting to access a nonexistent attribute results in an AttributeError
. However, by setting a default value as the third parameter in getattr()
, you can avoid errors and enhance code reliability. This is particularly useful in large-scale codebases or complex class structures.
getattr(user, 'address')
getattr(user, 'address', 'No address provided.')
Below is the result when executing line 1, which does not use a default value.

Accessing Methods
In addition to accessing an object’s attributes, getattr()
can also retrieve methods. You can access a method by name and then invoke it.
class Calculator:
def add(self, x, y):
return x + y
def subtract(self, x, y):
return x - y
calc = Calculator()
operation = 'add'
method = getattr(calc, operation)
method(5, 3)
operation = 'subtract'
method = getattr(calc, operation)
method(5, 3)
In this example, getattr()
is used to dynamically access the add
and subtract
methods, depending on the operation
variable. The output of method()
changes based on the value of operation
.
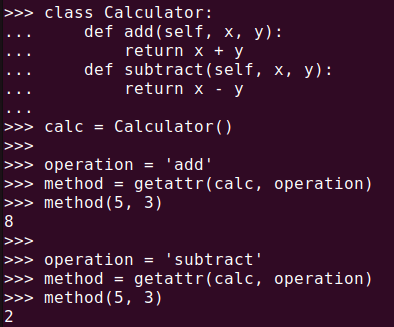
Precautions When Using the getattr() Function
- Error when accessing nonexistent attributes: When trying to access a non-existing attribute,
getattr()
will raise anAttributeError
unless you provide a default value as the third parameter. - Overuse of dynamic attribute access: Excessive use of
getattr()
can make your code difficult to maintain and complex. Use dynamic access only when necessary, and prefer static attribute access for most cases. - Security risks: When using
getattr()
with user input, you must exercise caution. Incorrect user input could allow access to unintended attributes, potentially leading to security vulnerabilities. Always validate user input thoroughly.
Summary
The getattr()
function is a powerful tool in Python that allows dynamic access to an object’s attributes and methods. It significantly increases flexibility when dynamic programming is required. However, excessive use can reduce code readability and maintainability, so it’s important to use it judiciously.
Additionally, setting default values to prevent errors and carefully handling user input are crucial for ensuring that your code runs securely and efficiently. When used properly, getattr()
can greatly enhance the flexibility and convenience of your Python code.