Python float()
function is used to convert other data types into floating-point numbers. Unlike integers, floating-point numbers can have decimal values. In this post, we will explore the basic usage of the float()
function and its various applications.
Table of Contents
What is Python float() Function?
The float()
function in Python converts string or integer data into a floating-point number. The basic syntax is as follows:
float(value)
Here, value
refers to the numeric or string data you wish to convert into a floating-point number. Utilizing floating-point numbers allows for precise calculations and is useful for various mathematical operations.
Basic Usage of the float() Function
Converting Integers to Floating-point Numbers
One of the simplest ways to use float()
is to convert integers into floating-point numbers.
num = 17
float_num = float(num)
print(float_num)
In this example, the integer 17
is converted into the floating-point number 17.0
using the float()
function. A key point to note is that when using float()
, a 0
is added after the decimal point, which ensures that Python recognizes the value as a floating-point number.
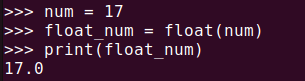
Converting Strings to Floating-point Numbers
The float()
function can also convert numeric strings into floating-point numbers.
num_str = "3.14"
float_num = float(num_str)
print(float_num)
In this example, the string "3.14"
is converted into a floating-point number. This is particularly useful when processing user input data, as input is often received as strings.
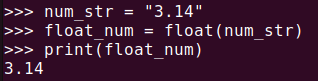
However, if a string cannot be converted to a number, an error will occur.
num_str = "hello"
float_num = float(num_str)
This will result in a “ValueError: could not convert string to float: ‘hello'” error message.

In such cases, you can use exception handling to manage the error:
try:
float_num = float("hello")
except ValueError:
print("Invalid value for conversion.")
As shown below, the print()
function inside the exception block is executed when the conversion fails.
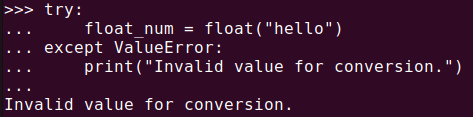
Converting Boolean Values to Floating-point Numbers
Boolean values such as True
or False
can also be converted into floating-point numbers. As shown in the figure below, True
is converted to 1.0
and False
is converted to 0.0
.
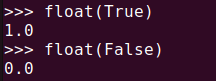
Handling Large and Small Numbers
The float()
function is useful for dealing with very large or very small numbers in Python. It handles these numbers using scientific notation.
large_num = 1e207
small_num = 1e-207
print(float(large_num))
print(float(small_num))
Here, 1e207
means 10^207, and the float()
function efficiently processes such large numbers.
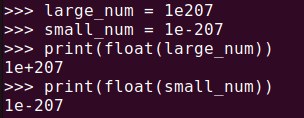
Dealing with NaN and Inf
The float()
function also handles special values like Not a Number (NaN) and Infinity (Inf). These are useful when dealing with abnormal calculation results.
nan_value = float("nan")
inf_value = float("inf")
print(nan_value)
print(inf_value)
NaN
represents an undefined or unrepresentable number, often occurring as a result of invalid calculations. Inf
stands for infinity, which can arise when dividing by zero or when dealing with extremely large numbers. You can also use Python’s math module to handle these values:
import math
nan_value = math.nan
inf_value = math.inf
Rounding with float()
When working with floating-point numbers, you often need to round them. Python allows you to adjust the number of decimal places using the round()
function.
num = 3.1415926535897
rounded_num = round(num, 2) # Rounds to 2 decimal places
print(rounded_num)
As shown below, the third decimal place and beyond are rounded to fit the second decimal place.

Arithmetic Operations with float()
The float()
function is frequently used in arithmetic operations. Python allows you to perform operations with both integers and floating-point numbers, and the result will automatically be converted into a floating-point number.
int_num = 10
float_num = 7.3
result = int_num + float_num
print(result)
As seen below, adding an integer and a floating-point number results in a floating-point number.
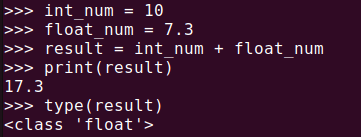
The float()
function is thus very useful in a wide range of arithmetic calculations.
Formatting Floating-point Output
When printing floating-point numbers, you can format the output to control the number of decimal places or the overall width of the output.
pi = 3.141592
print("%8.2f" % pi)
In the example below, the number is printed with two decimal places, taking up eight spaces in total.

Summary
Python’s float()
function is a powerful tool for converting numbers to floating-point format. It allows for precise calculations with large and small numbers, handles special values like NaN
and Inf
, and integrates with rounding and arithmetic operations. Additionally, when printing floating-point numbers, formatting options provide flexibility in how the results are displayed. When using float()
, it’s advisable to handle exceptions properly to ensure robust code.