The Python dict()
function is a useful tool for creating dictionaries. A dictionary stores data in key-value pairs, which is very useful for retrieving and processing data. In this post, we will explore various ways to use Python’s dict()
function.
Table of Contents
What is a Dictionary?
A dictionary in Python is a data structure similar to a “HashMap.” You can create a dictionary using curly braces {}
as shown below:
a = {"name": "Jack", "age": 38, "city": "Tokyo"}
In this code, name
, age
, and city
are the keys, while "Jack"
, 38
, and "Tokyo"
are the corresponding values. You can see a dictionary created with curly braces in the figure below.

Creating a Dictionary Using the dict() Function
The dict()
function is another way to create dictionaries. This method improves readability and is often used when keys and values are clearly defined. Here’s an example of creating a dictionary using dict()
:
b = dict(name="Jack", age=38, city="Tokyo")
This dictionary works the same way as the one created with curly braces {}
. The difference is that when using dict()
, the keys must be strings.

Creating a Dictionary Using the zip() Function
When the keys and values are in the form of lists, and both have the same number of elements, the zip()
function can be a convenient way to create a dictionary. The code below creates the same dictionary as before:
c = dict(zip(["name", "age", "city"], ["Jack", 38, "Tokyo"]))
Creating a Dictionary from a List of Tuples
If the data is structured as a list of tuples where each tuple contains a key-value pair, you can create a dictionary like this:
d = dict([("name", "Jack"), ("age", 38), ("city", "Tokyo")])
Creating a Dictionary Using Curly Braces
When passing a dictionary to the dict()
function, the order of the keys does not matter. You can use the dict()
function without worrying about the order of keys in the input dictionary or zip()
.
e = dict({"city": "Tokyo", "name": "Jack", "age": 38})
Combining Key-Value Methods to Create a Dictionary
You can also mix the use of curly braces and new key-value pairs when creating a dictionary.
f = dict({"name": "Jack", "city": "Tokyo"}, age=38)
In the figure below, you can see that all these methods create the same dictionary. The order of keys in a dictionary does not matter, so they all result in the same dictionary.
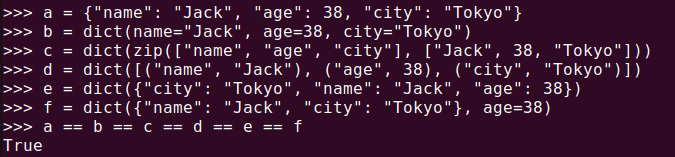
Important Notes When Creating Dictionaries
Creating a dictionary is simple, but there are a few things to keep in mind.
Keys Must Be Unique
The keys in a dictionary must be unique. If you create a dictionary using curly braces and the keys are duplicated, only the last value for that key will be stored. When using the dict()
function, Python will raise a SyntaxError
with a message like “SyntaxError: keyword argument repeated: variable_name” if the keys are duplicated.

Only Hashable Types Can Be Used as Keys
The keys in a dictionary can only be immutable types like strings or numbers. Mutable types like lists cannot be used as keys.

When Using dict(), Only Strings Can Be Used as Keys
Although dictionaries allow both strings and numbers as keys, when creating a dictionary using dict()
, only strings can be used as keys. In such cases, the error message is ‘SyntaxError: expression cannot contain assignment, perhaps you meant “==”?’. This typically occurs when you’ve accidentally used an assignment operator (=) where a comparison operator (==) was intended.

Summary
Python dictionaries (dict
) are a highly efficient way to store data as key-value pairs. When working with dictionaries, remember that keys must be unique and hashable. By leveraging these features, you can manage data more effectively in Python.