The Python delattr()
function is useful for dynamically deleting attributes from objects. In this post, we will explore the concept of the delattr()
function, its usage, and important considerations to keep in mind.
Table of Contents
What is the Python delattr() Function?
The delattr()
function is one of Python’s built-in functions, used to delete an attribute defined within an object. Here, an attribute refers to a variable or method that belongs to a class or object. Objects can have multiple attributes, and when certain attributes need to be removed, the delattr()
function is used.
delattr(object, 'attribute_name')
- object: Refers to the object whose attribute you want to delete.
- ‘attribute_name’: The name of the attribute you wish to delete, passed as a string.
Be aware that if the specified attribute does not exist, the delattr()
function raises an AttributeError
. Deleting attributes from an object is often useful when managing attributes dynamically or optimizing memory usage.
Basic Usage of delattr()
Let’s look at a basic example where we define a class, create an object, and use delattr()
to delete an attribute from that object.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person('Kimberly', 25)
print(person.name)
print(person.age)
delattr(person, 'age')
print(person.age)
As shown in the figure below, after using delattr()
to delete the age
attribute of the person
object, attempting to access this attribute results in an AttributeError
, indicating that the age
attribute no longer exists.
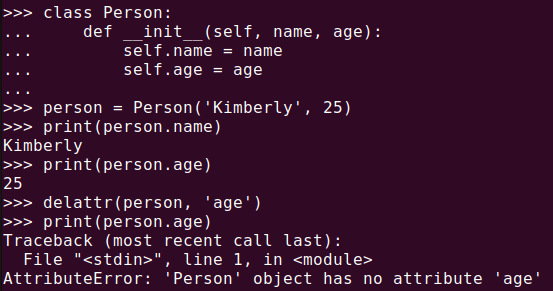
Conditional Attribute Deletion
You can write code that deletes an attribute only if it exists in the object. This approach helps prevent unexpected errors.
class Car:
def __init__(self, brand, model, year):
self.brand = brand
self.model = model
self.year = year
car = Car('Tesla', 'Model S', 2020)
if hasattr(car, 'year'):
delattr(car, 'year')
print(hasattr(car, 'year'))
In this code, the hasattr()
function is used to check if the year
attribute exists in the object. The attribute is only deleted if it exists, thus avoiding any potential errors.
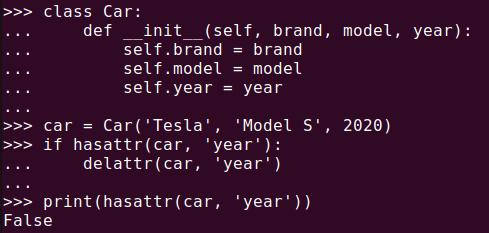
Important Considerations
There are several important considerations when using delattr()
.
- Error When Deleting Non-Existent Attributes
If you try to delete an attribute that does not exist, delattr() will raise an AttributeError. Therefore, it’s essential to verify that the attribute exists before attempting to delete it. - Be Cautious of Deleting Built-in Attributes
Python objects have important built-in attributes like__init__
and__str__
. If these attributes are accidentally deleted, it can disrupt the normal behavior of the object. Therefore, caution is required when deleting attributes. - Dynamically Added Attributes
Dynamically added attributes can also be deleted withdelattr()
. However, when using this approach, make sure to properly manage the presence or absence of such attributes throughout your code to avoid issues when the deleted attributes are accessed later.
Summary
The Python delattr()
function is a useful tool for dynamically deleting object attributes. Deleting attributes conditionally is a safer way to use this function. However, you should be cautious when deleting non-existent attributes, as it may cause errors, and you should avoid deleting important built-in attributes.
By using this function appropriately, you can increase the flexibility of Python objects and improve memory efficiency. Try applying the delattr()
function in various scenarios to manage your Python objects more effectively!