The Python classmethod() is a useful feature for defining class methods. In Python, you often come across concepts related to object-oriented programming, such as handling classes and methods. In this post, we’ll explore the concept and usage of classmethod()
in detail.
Table of Contents
What is Python classmethod()?
The Python classmethod() is an important function for handling classes. Typically, methods defined within a class are instance methods, meaning they take the instance of the object as the first argument. However, the classmethod()
is used to define methods that take the class itself as the first argument.
Such class methods perform operations related to the entire class rather than a specific object, making them useful for accessing class variables or performing class-level tasks.
How to Use classmethod()
Basic Syntax
Here is the basic syntax for using Python classmethod()
:
class class_name:
@classmethod
def method_name(cls, arguments):
pass
Here, cls
is a parameter representing the class itself, and it is used in place of self
. With cls
, you can access class variables or perform operations related to the class itself.
Example: Managing Data at the Class Level
With classmethod()
, you can efficiently manage or update data at the class level. Let’s take a look at an example:
class Counter:
count = 0 # class variable
@classmethod
def increase(cls):
cls.count += 1
@classmethod
def get_current(cls):
return cls.count
In this example, the Counter
class has a class variable count
, and every time the increase()
method is called, the value of the class variable increases by 1. The key point here is that the method affects the entire class, not just a specific object.
Since this is a class method and not an instance method, it is manipulated directly at the class level. Even if instances are created, the variable remains the same at the class level and does not get reset within instances. The image below helps illustrate this concept.
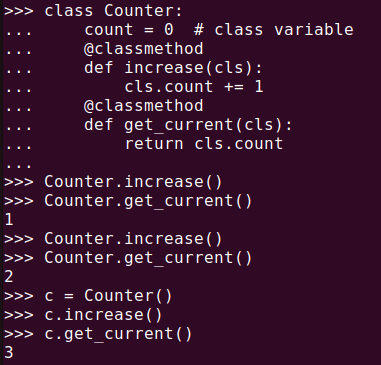
Difference Between Class Methods and Instance Methods
It is essential to understand the difference between classmethod()
and regular instance methods. Instance methods take the instance of the object as the first argument and can access instance variables. In contrast, class methods take the class itself as the first argument and can only access class variables.
class Sample:
instance_variable = 0
class_variable = 0
def instance_method(self):
self.instance_variable += 1
@classmethod
def class_method(cls):
cls.class_variable += 1
In the code above, the instance_method()
accesses the object’s instance variable, while the class_method()
affects only the class variable. This clearly demonstrates the difference between methods operating at the instance and class levels.
Also, you cannot access instance methods directly from the class since they require self
as the first argument, representing the object itself. Attempting to do so will result in an error like: “TypeError: Sample.instance_method() missing 1 required positional argument: ‘self'”.
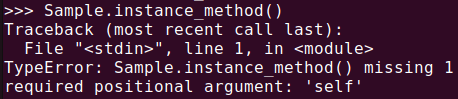
Examples Where classmethod() Is Particularly Useful
Managing Database Connections
classmethod()
can be useful when maintaining database connection information at the class level, allowing multiple instances to share the same connection. This makes resource management more efficient as the same connection can be reused across different instances.
class Database:
conn = None
@classmethod
def connect(cls, user_id, user_pw):
cls.conn = connect_to_sql(user_id, user_pw)
@classmethod
def get_conn(cls):
return cls.conn
Database.connect("user", "pass")
In this code, the database connection is managed through a class variable, and all instances can share this connection. This approach eliminates the need to create multiple connections, optimizing resource management.
Modifying Behavior of Inherited Classes
classmethod()
is also helpful when you want to redefine class methods or modify class variables in inherited classes.
class Animal:
name = "Animal"
@classmethod
def get_name(cls):
return cls.name
class Dog(Animal):
name = "Dog"
class Cat(Animal):
name = "Cat"
print(Dog.get_name())
print(Cat.get_name())
In this example, the Dog
and Cat
classes inherit from the Animal
class, but each subclass returns its own name
through the class method.
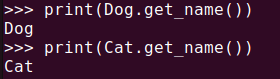
Cautions When Using classmethod()
- Class Variables vs. Instance Variables:
classmethod()
can only access class variables, not instance variables. Therefore, it is not suitable for tasks related to an individual object’s state. - Avoid Using
self
in Class Methods: Since class methods operate on the class itself, they usecls
rather thanself
. The key difference here is that class methods take the class itself as the first argument, not an instance. - Understanding Method Behavior During Inheritance: Class methods will work the same way in inherited classes, but they will be called within the context of the child class. This can lead to unintended behavior if you’re not careful. Ensure you fully understand how class methods behave in an inheritance structure before using them.
- Avoid Unnecessary Use: Not all methods should be class methods. If an instance method is more appropriate for your use case, avoid using class methods unnecessarily. Only use class methods when required.
- Memory Management Considerations: Since class methods manage data at the class level, improper usage could lead to memory leaks or unexpected state changes. Handle class variables carefully and modify them only when necessary.
Summary
Python classmethod() is a valuable method when performing tasks at the class level. It can be useful for implementing factory methods, managing class variables, and handling tasks that apply to the entire class rather than specific instances. However, it is important to avoid confusing it with instance methods and to use it in appropriate situations. If you want to deepen your understanding of Python’s object-oriented programming, mastering the principles and applications of classmethod()
is essential.