The Python chr()
function is a useful feature that converts character codes (ASCII codes or Unicode code points) into their corresponding characters. It is especially handy when you need to handle characters or print special characters in Python. In this post, we will explore the basic usage of the chr()
function and its applications.
Table of Contents
What is the Python chr() function?
The Python chr()
function takes an integer and returns the character corresponding to that number. The input integer can be an ASCII code (American Standard Code for Information Interchange) or a Unicode value.
For example, in ASCII, the number 97 corresponds to the character ‘a’, and 65 corresponds to the character ‘A’. Using the chr()
function, you can convert these numbers into their respective characters.
Converting ASCII codes to characters
The following is an example of converting ASCII codes to characters using the chr()
function.
Pythonchr(97)
chr(65)
In the figure below, the number 97 is converted to ‘a’, and 65 is converted to ‘A’. In this way, you can convert various numbers into characters.
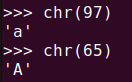
Converting Unicode to characters
The chr()
function supports not only ASCII codes but also Unicode. Unicode is an international standard for representing characters from around the world. Since Unicode includes characters from various languages, the chr()
function can be used to display Korean characters, emojis, and more.
For example, in Unicode, 12354 corresponds to the Japanese character ‘あ’, 29233 corresponds to the Chinese character ‘爱’, and 44032 corresponds to the Korean character ‘가’. You can convert these using the chr()
function as follows:
chr(12354)
chr(29233)
chr(44032)
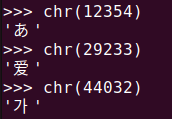
Additionally, the chr()
function can be used to print special characters like emojis. For example, the emoji ‘😊’ corresponds to Unicode 128522. This is especially useful when inserting emojis into social media posts or web pages. If you know the Unicode value of an emoji, you can easily display it.
chr(128522)
chr(128515)
chr(128516)
chr(128517)
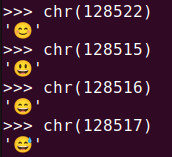
Useful applications of the chr() function
Using the Python ord() function together
In Python, the ord()
function can be used alongside chr()
to convert characters to ASCII codes and back. For example, you can convert a character to its ASCII code and then convert it back to the character using chr()
.
ascii_code = ord('A')
print(ascii_code)
chr(ascii_code)
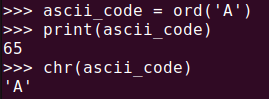
This approach allows you to easily convert a character if you know or calculate its ASCII code.
Printing special characters
The chr()
function is very useful when printing special characters. For example, you can output a newline character '\n'
using its ASCII code or check a tab character '\t'
through the code.
chr(10)
chr(9)
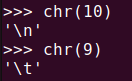
You can experiment with and use various special characters by converting them through code.
Precautions: Entering numbers outside the valid range
If you input an invalid number into the chr()
function, it will result in an error. For instance, if you input a number outside the valid ASCII code range (e.g., a negative number), a ValueError
will occur.
try:
print(chr(-1))
except ValueError as e:
print(f"Ocurred Error: {e}")
When using the chr()
function, always ensure that the input number is within the valid range. As shown in the “ValueError: chr() arg not in range
(0x110000)”, the valid range is between 0 and 1,114,111 in Unicode (hexadecimal 0x110000).
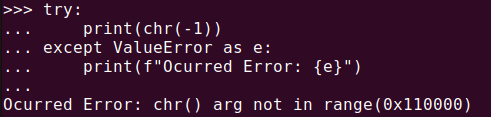
Summary of chr() usage
- The
chr()
function is a Python built-in function that converts integers into characters. - It supports both ASCII codes and Unicode, allowing you to print a wide range of characters.
- You can use the
ord()
function in conjunction withchr()
to convert between ASCII codes and characters. - It is helpful for printing special characters and emojis.
- Ensure that the input number is within the valid range, which is between 0 and 1,114,111.
Summary
The chr()
function in Python provides a simple yet highly useful way to convert integers into characters. It works with both ASCII and Unicode characters, enabling you to output a variety of characters and emojis. It’s also handy for handling special characters. Just be mindful of the valid input range when using the chr()
function. This method, particularly with Unicode emojis, can be creatively applied in fields such as social media development.