Using the Python bytearray()
function allows for efficient manipulation of mutable binary data while maintaining the properties of a byte array. In other words, you can manipulate binary data just like strings or lists. In this post, we’ll explore how to use the bytearray()
function, its applications, and the important things to keep in mind.
Table of Contents
What is Python bytearray()?
bytearray()
is a variable-length sequence of bytes provided by Python. A byte array essentially handles binary data and can be manipulated like strings. The key difference between bytearray()
and bytes
is that bytearray()
is mutable, meaning you can change its contents.
Basic Usage of bytearray()
You can create a bytearray()
in several ways. The simplest method is to create an empty byte array:
b = bytearray()
print(b)
In addition to an empty array, you can also convert other data types into a byte array:
b = bytearray("Hello", "utf-8")
print(b)
b = bytearray([72, 101, 108, 108, 111])
print(b)
Converting the string “Hello” into a byte array or converting a list of ASCII values into bytearray(b'Hello')
results in the following:
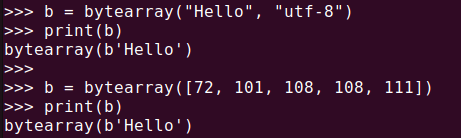
As shown, bytearray()
can accept various data types as input and convert each into a byte array.
Manipulating a Byte Array
Since bytearray()
is mutable, you can change its contents. For example, you can modify or append values to specific indices of the byte array.
Modifying Byte Array Values
You can change the value at a specific index, similar to how you would with a list. However, note that each value must be an integer between 0 and 255.
b = bytearray(b'Hello')
b[1] = 97
print(b)
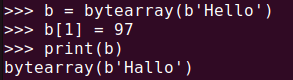
Adding Values to a Byte Array
Just like lists, you can use the append()
function to add a value to the end of a byte array:
b.append(33)
print(b)
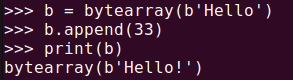
You can also add multiple values at once using the extend()
function:
b.extend([32, 87, 111, 114, 108, 100])
print(b)

Converting Between Strings and Byte Arrays
Since bytearray()
primarily handles binary data, converting between strings and byte arrays is essential. You can use the decode()
and encode()
methods for this purpose.
Converting a Byte Array to a String
To convert a byte array to a string, use decode()
. Be sure to specify the encoding format, with ‘utf-8’ being the most common.
b = bytearray(b'Hello')
s = b.decode('utf-8')
print(s)
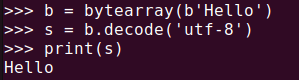
Converting a String to a Byte Array
To convert a string into a byte array by encoding it in ‘utf-8’, use encode()
:
s = "Hello"
b = bytearray(s.encode('utf-8'))
print(b)
Caution: Byte Range
Each element in a bytearray()
must be a value between 0 and 255. Attempting to add or modify a value outside this range will result in an error.
b = bytearray(b'Hello')
b[0] = 300
This results in the “ValueError: byte must be in range(0, 256)” error, reminding you to use values within the 0–255 range. Always ensure values remain within this range when working with byte arrays.
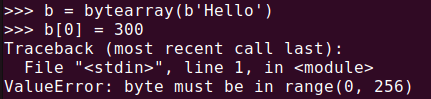
Application of bytearray()
The bytearray()
function is particularly useful when handling binary data in network communication or file I/O. For example, it is often used when processing data received from a network socket or when reading and writing binary files.
Reading Files with Byte Arrays
You can use bytearray()
to process data when reading binary files:
with open("example.bin", "rb") as file:
data = bytearray(file.read())
print(data)
In situations where binary files need to be handled, the mutable nature of bytearray()
allows for efficient data manipulation.
Summary
The Python bytearray()
function is a powerful tool for handling mutable binary data. While offering the ability to manipulate data like a string, it retains the characteristics of a byte array, providing high memory efficiency. It’s especially useful in networking and file I/O scenarios. As long as you keep in mind the byte range limitations, bytearray()
can be utilized in various applications to process binary data more efficiently.
Consider making use of bytearray()
for efficient binary data processing.