The Python bool()
function is an essential tool for determining whether a given value is True
or False
. The bool()
function can be applied to various data types and values, and it returns a boolean result of either True
or False
. In this post, we will explore the basic usage of the bool()
function, how it can be applied in real-world development, and some important caveats to keep in mind.
Table of Contents
Basic Concept of the bool()
Function
The bool()
function is a built-in Python function that determines whether a given value is True
or False
. These are logical values, which Python represents as True
and False
.
Syntax:
bool(value)
Here, “value” refers to the object whose truthiness is being checked by the bool()
function. All values can be converted to either True
or False
, and Python treats certain values as False
by default.
Values Treated as False
The following values in Python are always evaluated as False
when passed to the bool()
function:
- Number 0 (
0
,0.0
,0j
, etc.) - Empty strings (
""
) - Empty lists (
[]
), empty tuples (()
), empty dictionaries ({}
), empty sets (set()
) - The value
None
(meaning no value) - The
False
value itself
All other values are treated as True
by default. For example, any non-zero number, non-empty string, or non-empty list or tuple will return True
when passed to the bool()
function.
print(bool(0))
print(bool(""))
print(bool([]))
print(bool(None))
print(bool(10))
print(bool("Python"))
print(bool([1, 2, 3]))
As seen above, 0
, an empty string, an empty list, and None
all evaluate to False
. On the other hand, values like 10
or the string “Python” are evaluated as True
.
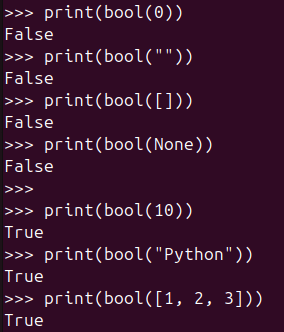
bool()
function results: True and FalseUse Cases of the bool()
Function
Using bool()
in Conditional Statements
The bool()
function is frequently used in conditional statements. These statements often involve checking whether a value is True
or False
, and bool()
can be applied directly or the value itself can be evaluated.
For example, you can use bool()
to check whether a list is empty:
my_list = []
if bool(my_list):
print("The list contains values.")
else:
print("The list is empty.")
However, when used in conditional statements, bool()
can be omitted for simplicity:
if my_list:
print("The list contains values.")
else:
print("The list is empty.")
Validating Data with bool()
The bool()
function is also useful for validating whether user input is valid. For instance, it can easily check if the input provided by a user is empty.
user_input = input("Enter a value: ")
if bool(user_input):
print("You entered:", user_input)
else:
print("No value was entered.")
In this case, if the input is an empty string, bool(user_input)
will return False
, and the message “No value was entered” will be printed. If any value is entered, True
will be returned, and the input will be displayed.
In the following illustration, if the string “0” is entered, it will be treated as a value, so “0” will be printed. If the Enter key is pressed without any input, the message “No value was entered” will appear.
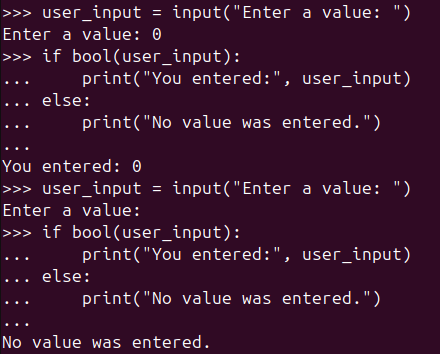
bool()
functionSimplifying Function Return Value Checks
The bool()
function can also simplify the evaluation of a function’s return value. For example, it can be used to determine whether an operation was successful.
def operation_success():
return []
result = operation_success()
if bool(result):
print("The operation was successful.")
else:
print("The operation failed.")
In this case, since the function returns an empty list, bool(result)
evaluates to False
, and the message “The operation failed” is printed. Although you could use len()
to check the length of the list, using bool()
provides a simpler and more concise solution.
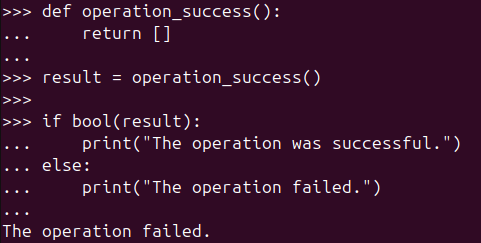
bool()
functionImportant Considerations
Misunderstandings with Numbers and Strings
All numbers except for 0
are considered True
. For instance, even negative numbers such as -1
are evaluated as True
. However, the string "0"
is treated as True
because it is a non-empty string. If you want the string "0"
to be treated as 0
, you need to use the int()
function to convert it.
print(bool(-1))
print(bool("0"))
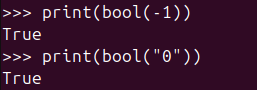
bool()
functionEmpty Containers
Lists, tuples, dictionaries, and sets are all considered False
if they are empty, but they will evaluate to True
as long as they contain at least one item.
print(bool([0]))
print(bool({}))
In the first line, the list contains the value 0
, so it evaluates as True
. In the second line, the dictionary is empty, so it evaluates as False
.
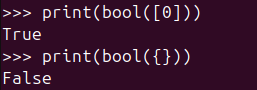
bool()
functionNone
None
is a special value in Python that represents the absence of a value. When evaluated by the bool()
function, None
returns False
. It is commonly used to represent initial states or error conditions.
print(bool(None))
Summary
The Python bool()
function is a powerful tool for easily checking whether a value is True
or False
. Whether you are working with conditional statements, validating data, or evaluating function return values, bool()
simplifies the process. However, it is essential to be aware of special cases such as numbers, empty containers, and the None
value.
Thanks to Python’s flexible type conversion, the bool()
function can be applied in various scenarios, improving the readability of your code and helping to avoid errors. The next time you write a program, make sure to consider using bool()
to create more efficient and clear code.