The Python bin()
built-in function is used to convert an integer into a binary string. This function is particularly useful when you need to perform operations that require binary conversions, such as bitwise operations, visualizing binary representations, or analyzing specific bit patterns. In this post, we’ll delve into the basic usage of the bin()
function and explore several examples to demonstrate its applications.
Table of Contents
Basic Usage of Python bin() Function
The bin()
function takes an integer as its argument and returns a string representing that integer in binary form. The returned string always begins with ‘0b’, which indicates that the value is in binary.
number = 10
string_binary = bin(number)
print(string_binary)
In the example above, converting the integer 10 to binary using the bin()
function results in the string '0b1010'
, as shown in the figure below. The ‘0b’ prefix indicates that Python recognizes this as a binary number.
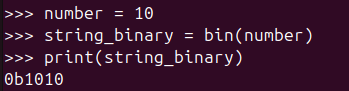
Practical Examples of Using the bin()
Function
Example 1: Counting the Number of Bits in a Binary Number
You can use the bin()
function to count the number of specific bits (e.g., 1s) in a binary number.
number = 29 # In binary: 11101
string_binary = bin(number)
count_of_ones = string_binary.count('1')
print(f"Number of 1s in the binary representation of {number}: {count_of_ones}")
As shown in the figure below, you can verify the number of 1s in the binary representation.

bin()
functionExample 2: Bitwise Operations Between Two Integers
You can also use the bin()
function to display the result of bitwise operations between two integers.
a = 15
b = 8
# Binary representation of the XOR operation result
xor_result = bin(a ^ b)
print(f"""a: {bin(a)}
b: {bin(b)}
------------
XOR: {xor_result}""")
The figure below shows the XOR result of a
and b
, which is 0b111
.
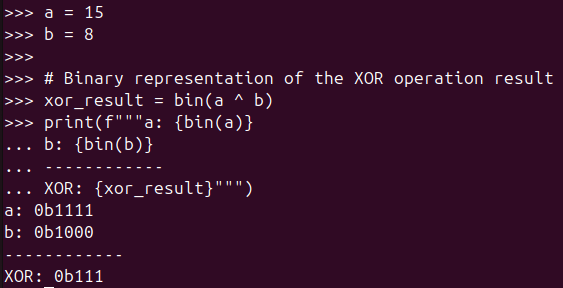
bin()
functionExample 3: Bit Masking with Binary Numbers
Bit masking allows you to filter or extract specific bits from a number. For instance, here’s how to extract the lower 4 bits from an integer.
number = 29 # In binary: 11101
mask = 0b1111 # Mask for extracting the lower 4 bits (binary: 1111)
result = number & mask
print(f"Lower 4 bits of {number}: {bin(result)}")
The bit masking result is displayed in the figure below.

bin()
functionSummary
The Python bin()
built-in function is a powerful tool for easily converting integers into binary strings. This capability simplifies various tasks related to bitwise operations and binary number manipulation. By practicing with the examples above, you can become proficient in leveraging the bin()
function whenever the need arises.