The Python abs()
built-in function is a simple yet useful tool that returns the absolute value of a number. In this post, we’ll explore how the abs()
function works, provide usage examples, and discuss practical applications.
Table of Contents
What is Python abs()
Built-in Function?
The abs()
function is one of Python’s built-in functions that returns the absolute value of a number. The absolute value is the non-negative value of the number, stripping away any sign (positive or negative). For example, the absolute value of -3
is 3
, and the absolute value of 3
is also 3
.
Basic Syntax of the abs()
Function
The basic syntax of the abs()
function is as follows:
abs(number)
- number: The number for which you want to find the absolute value. This number can be an integer, a floating-point number (float), or a complex number (complex).
The function returns the absolute value of the input number.
Executing from Objects
While the built-in abs()
function is commonly used, it can also be implemented as a method within a value object.
num = -3
num.__abs__()
Usage Examples
Using with Integers
When you pass an integer as a parameter, the function returns the non-negative version of that integer.
print(abs(-10))
print(abs(5))
As shown in the figure below, -10 is output as 10, and 5 remains as 5.
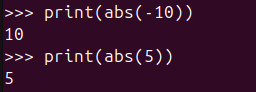
abs()
function to integersUsing with Floating-Point Numbers
For floating-point numbers, the absolute value is returned similarly as a non-negative floating-point number.
print(abs(-3.14))
print(abs(0.0))
As shown in the figure below, -3.14 is output as 3.14, and 0.0 remains as 0.0.
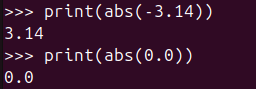
abs()
function to floating-point numbersUsing with Complex Numbers
In Python, complex numbers consist of a real part and an imaginary part. The abs()
function returns the magnitude (absolute value) of the complex number. The magnitude is calculated using the Pythagorean theorem.
complex_number = 3 + 4j
print(abs(complex_number)) # (sqrt(3^2 + 4^2) = 5)
As shown below, the absolute value is also output for complex numbers.
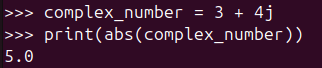
abs()
function to complex numbersReal-World Applications of the abs()
Function
The abs()
function can be useful in various situations, such as calculating the distance between two points or handling negative values.
Calculating the Distance Between Two Points
The distance d
between two points (x1, y1)
and (x2, y2)
on a plane can be calculated as follows:
import math
x1, y1 = 0, 0
x2, y2 = 3, 4
distance = math.sqrt(abs(x2 - x1)**2 + abs(y2 - y1)**2)
print(distance)
Below is the result of calculating the distance between the two points.
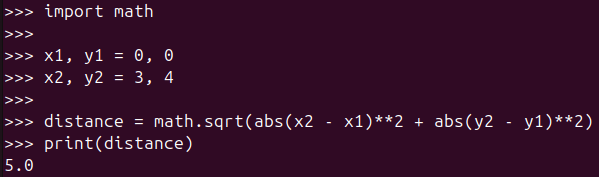
abs()
function for distance calculationHandling Negative Values
The abs()
function is also helpful when you want to remove negative values during data processing. For example, you can use it to calculate the magnitude of price changes when analyzing stock price fluctuations.
price_change = -7.25
print(abs(price_change))
Summary
Python’s abs()
function is incredibly simple but can be a powerful tool in a variety of situations. Whenever you need to find the absolute value of a number, this function is your go-to solution. By understanding how to use this function and applying it to various cases, you’ll be able to write more efficient and intuitive code. This post has covered the basics of Python’s abs()
function, including usage examples and real-world applications. We hope this guide has been helpful!