In this post, we’ll explore how to install Python packages using the pip install
command, along with several useful options. Python is a programming language rich in libraries that allow for easy implementation of various functionalities. These libraries are provided in the form of packages, which developers can readily use. We’ll dive into how to use the pip install
command—a primary tool for installing and managing these packages—and its related options.
Table of Contents
What is pip?
pip
stands for “Python Package Index” and is a package manager used to install and manage Python packages. In a Python development environment, pip
is an essential tool that allows you to easily install a wide range of publicly available libraries and packages from the internet.
Basic Installation Method with pip install
The most fundamental command is pip install
. This command enables you to easily install the desired package. For instance, to install the numpy
package, which is widely used for data analysis, you would enter the following command:
pip install numpy
When you execute the command above, pip
will locate, download, and install the numpy
package from the internet. This simple command automatically handles the complex installation process.
Installing Multiple Packages at Once
If you want to install multiple packages at once, you can list the package names separated by spaces. For example, to install three packages—numpy
, pandas
, and matplotlib
—at the same time, you can enter the following:
pip install numpy pandas matplotlib
Executing this command will install all three packages sequentially. Installing multiple packages simultaneously can save time and prepare the essential libraries for your project all at once.
Various Installation Options with pip install
pip install
comes with several useful options. These options allow you to install specific versions, check the information of installed packages, and perform other tasks.
Installing a Specific Version
Sometimes, you may need to install a specific version of a package instead of the latest one. For example, if you want to install version 1.2.0 of the pandas
package, you can use the following command:
pip install pandas==1.2.0
By entering the desired version number after the ==
symbol, you can install that particular version of the package. This feature is useful for projects that work best with specific package versions or to resolve compatibility issues.
–upgrade: Upgrading to the Latest Version
If you want to upgrade an installed package to the latest version, use the --upgrade
option. For example, to upgrade the requests
package to its latest version, enter the following command:
pip install --upgrade requests
This command checks the current version of the installed package and automatically upgrades it if a newer version is available.
–no-deps: Ignoring Dependencies
Python packages often depend on other packages. For example, installing matplotlib
may require numpy
. pip
automatically handles these dependencies by installing the required packages along with the specified one. However, if you need to resolve specific dependency issues or install only certain versions of dependencies, you can use the --no-deps
option:
pip install matplotlib --no-deps
This option installs only the specified package without its dependencies, which can cause problems by breaking essential dependency management. Therefore, this option should be used with caution when you need to manually manage dependencies.
-r: Installing from a requirements.txt File
If you want to install the same set of packages as in another environment, you can save the installed packages into a requirements file and install them using the requirements.txt
file. To install packages from a requirements.txt
file, use the following command:
pip install -r requirements.txt
This command installs all the packages listed in the requirements.txt
file at once, making it easy to replicate the development environment.
Checking and Managing Installed Packages
You can also check and manage installed packages through pip
. This allows you to verify which packages are installed in your current environment and remove unnecessary ones.
Checking the List of Installed Packages
To check the list of currently installed packages, use the pip list
command:
pip list
This command outputs all the packages installed in your current environment along with their versions. It helps you easily verify the status of installed packages. Below, you can see an example of the package names and versions installed.
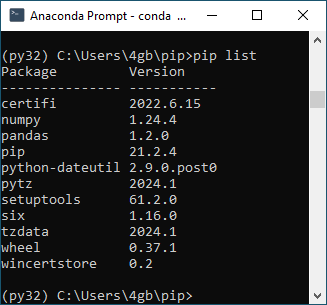
Uninstalling Packages
If you no longer need a package, you can remove it using the pip uninstall
command. For example, to uninstall the numpy
package, enter the following command:
pip uninstall numpy
When you enter this command, pip
will remove the specified package from your system. A confirmation prompt will appear, and once you enter “y”, the uninstallation will be completed.
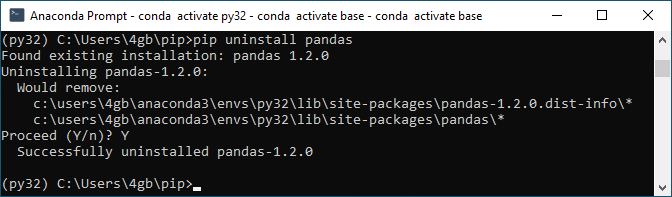
Checking Information of Installed Packages
If you want to check detailed information about a specific installed package, you can use the pip show
command. For example, to check information about the requests
package, use the following command:
pip show numpy
This command provides information about the package’s version, installation location, dependencies, and more.
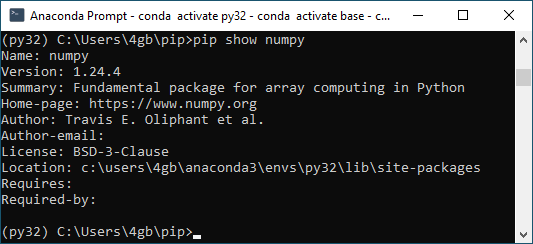
Important Notes
There are a few important considerations when using the pip install
command. First, it is advisable to avoid installing packages across the entire system with root privileges. Instead, it is recommended to use a virtual environment. A virtual environment provides an isolated Python environment for each project, preventing conflicts between packages and maintaining a cleaner development environment.
Also, keep in mind that the latest version is not always the best option. Some projects may only work stably with specific versions, so managing versions carefully is crucial.
Summary
pip install
is a crucial tool in Python development, offering powerful features for package installation and management. In this post, we covered the basics of using the pip install
command, various options, package management methods, and some hidden useful features. By using pip
correctly, you can efficiently manage your Python development environment and easily install the necessary packages whenever needed. As you continue working on Python projects, make good use of the knowledge gained from this post.