Python hex()
function is a powerful tool that allows you to easily convert and utilize hexadecimal (base-16) numbers. Being able to represent numbers in various formats is quite useful in programming, and hexadecimal is a particularly important concept, especially when dealing with internal data processing in computers. In this post, we’ll explain how to use the hex()
function and its various applications in a simple manner.
Table of Contents
What is Python hex() Function?
The hex()
function is a built-in function in Python that converts integers into hexadecimal string representations. Hexadecimal is a numbering system that uses 16 characters: the numbers 0-9 and the letters A-F. Unlike decimal, which uses base 10, hexadecimal is base 16, which means it can represent larger numbers with fewer digits, making it useful for data processing and memory address representation.
Basic Usage of hex()
The hex()
function serves the straightforward purpose of converting an integer into its hexadecimal form. The result is returned as a string but can be internally recognized as a hexadecimal number. Here’s how you can convert a number to hexadecimal in Python:
number = 255
hex_number = hex(number)
print(hex_number)
In the code above, the number 255 is converted into its hexadecimal equivalent, which is 0xff
. The 0x
prefix indicates that the number is in hexadecimal format.
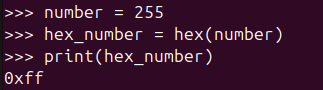
Negative Number Conversion
In Python, if you convert a negative number using the hex()
function, the negative sign is preserved in the hexadecimal output.
n = -42
hex_value = hex(n)
print(hex_value)
In this example, -42 is converted to its hexadecimal representation -0x2a
. As you can see, the 0x
prefix remains while the negative sign is retained.
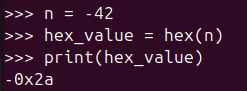
Combining with int() Function
While the hex()
function converts numbers to hexadecimal, you can convert hexadecimal strings back into integers using the int()
function. By passing 16 as the second argument, you can specify that the string is in hexadecimal format and have it converted back to decimal.
hex_value = '0xff'
decimal_value = int(hex_value, 16)
print(decimal_value)
In this code, the hexadecimal string 0xff
is converted back into its decimal equivalent, which is 255.
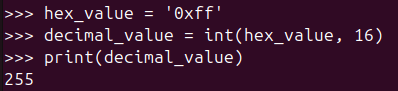
Hexadecimal Output Without hex(): Using String Formatting
Python also allows for more fine-grained control over hexadecimal output through string formatting. By using the format()
function or f-strings, you can print hexadecimal numbers without relying on the hex()
function.
n = 255
format(n, 'x')
format(n, '#x')
formatted_hex = f'{n:#x}'
print(formatted_hex)
formatted_hex_no_prefix = f'{n:x}'
print(formatted_hex_no_prefix)
This approach enables you to control whether or not to include the 0x
prefix when displaying the hexadecimal value.
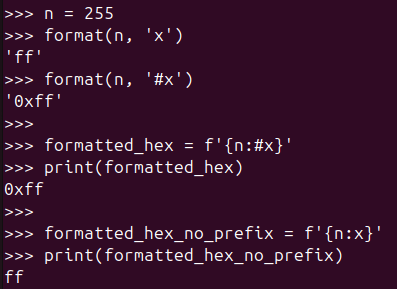
Uppercase Hexadecimal Output
By default, hexadecimal numbers output by the hex()
function are in lowercase. If you’d prefer uppercase, you can use the upper()
method.
n = 255
hex_value = hex(n).upper()
print(hex_value)
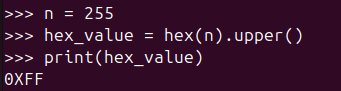
You can also use f-strings or format()
to directly print uppercase hexadecimal values:
n = 255
formatted_hex = f'{n:#X}'
print(formatted_hex)
format(n, '#X')
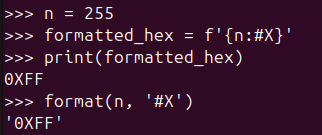
Real-World Uses of Hexadecimal
Hexadecimal is commonly used in computer science for representing memory addresses or defining color codes. For example, in web development, colors are often specified in hexadecimal, combining the RGB (Red, Green, Blue) values into a single hexadecimal value.
<div style="color: #FF5733;">Hello World</div>
In addition, hexadecimal plays an important role in low-level programming and memory management. For instance, in Linux, the xxd
command allows you to view the contents of a file in hexadecimal format, which is helpful for debugging or analyzing raw data.

Things to Watch Out for When Using hex()
There are a few things to keep in mind when using the hex()
function:
- String vs. Integer Confusion: The
hex()
function converts integers to hexadecimal strings, so if you need to use the result as a number, you will have to convert it back to an integer usingint()
. - Floating Point Numbers: The
hex()
function cannot directly convert floating-point (decimal) numbers. If you try to usehex()
on a float, you’ll need to first convert it to an integer.
float_number = 3.14
hex(float_number)
In cases like the one above, you need to convert the float to an integer before using hex()
. If you don’t, Python will raise a “TypeError: ‘float’ object cannot be interpreted as an integer.”

Summary
In this post, we explored how to use Python hex()
function to convert decimal numbers into hexadecimal. The hex()
function is a simple yet essential tool in computer programming, allowing you to handle data more efficiently in contexts such as color codes or memory addresses. Moreover, by combining it with the int()
function or using string formatting, you can achieve greater flexibility in how you handle hexadecimal numbers.
Whether you’re working with low-level data processing or system programming, the hex()
function provides a quick and convenient way to convert numbers into hexadecimal.