In this article, we will explain how to create a Python virtual environment and manage packages using pip. When working on various projects in Python, it is crucial to manage libraries or packages in a way that prevents them from conflicting with each other. To solve this problem, Python offers a solution called a Virtual Environment. A virtual environment allows each project to have its independent environment, ensuring that libraries used in one project do not affect another.
Table of Contents
What is a Python Virtual Environment?
A virtual environment is an isolated Python runtime environment dedicated to a specific project. For example, if you have two projects that require different versions of the same library, a virtual environment allows you to create independent environments for each project, preventing any conflicts.
Why You Need a Virtual Environment
Using a virtual environment provides the following benefits:
- Prevents library conflicts between projects: It allows you to avoid conflicts when different projects require different versions of the same library.
- Ensures project reproducibility: By specifying the exact version of the libraries needed for a specific project, you can recreate the same environment on another system.
- Protects the system environment: You can install libraries needed only for a specific project without affecting the global environment.
Creating a Virtual Environment with pip and venv
pip
is a tool used to install and manage packages in Python. venv
is a built-in Python module that makes it easy to create and manage virtual environments. Now, let’s look at how to create a virtual environment using pip and venv.
Creating a Virtual Environment
Check Python Installation: First, make sure that Python is installed. You can check the version by entering the following command in your terminal or command prompt.
python --version
Create a Virtual Environment: Navigate to your project directory and create a virtual environment with the following command. Here, myenv
is the name of the virtual environment, which you can change as desired.
python -m venv myenv
This command creates a directory named myenv
in the current directory and sets up an independent Python environment within it. Inside the myenv
directory, you will find directories and files necessary for the virtual environment.
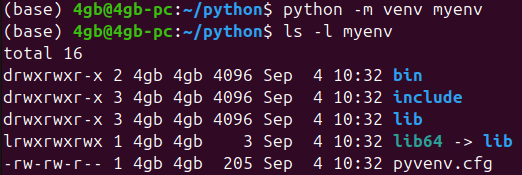
Activating and Deactivating the Virtual Environment
When you activate the virtual environment, any libraries you install or use will be confined to that environment.
Activating the Virtual Environment
The activation command varies depending on your operating system.
Windows
To activate the Python virtual environment on Windows, enter the following:
myenv\Scripts\activate
Once activated, you will see the virtual environment name appear at the beginning of the command prompt, as shown below.

MacOS/Linux
To activate the Python virtual environment on MacOS/Linux, enter the following:
source myenv/bin/activate
Once activated, the virtual environment name will appear in the terminal prompt, indicating that the environment is active, as shown below.

Deactivating the Virtual Environment
If you want to exit the virtual environment, simply enter the following deactivate
command, regardless of your operating system. You will then return to your system’s default Python environment, as shown below.

Installing and Managing Packages with pip
Once the virtual environment is activated, you can install and manage packages using pip.
Installing Packages
To install a package, use the pip install
command. For example, to install a package called requests
, enter the following:
pip install requests
This command installs the requests
package within the virtual environment. The installed package is stored in the lib\site-packages
directory of the virtual environment. For more detailed usage of pip install
, refer to the article “Installing Python Packages: pip install and 3 Key Options.”
Viewing Installed Packages
To see a list of packages installed in the virtual environment, use the following command:
pip list
This command displays a list of all packages currently installed in the virtual environment. For more detailed usage of pip list
, refer to the article “How to Use pip list and 4 Options.”
Uninstalling Packages
To uninstall a package that is no longer needed, use the pip uninstall
command. For example, to uninstall the requests
package, enter the following:
pip uninstall requests
Using the requirements.txt File
When managing multiple packages, it is useful to use a requirements.txt
file. This file lists all the packages and their versions required for the project, allowing others to easily recreate the same environment.
Creating a requirements.txt File
pip list --format=freeze > requirements.txt
This command saves all the packages installed in the current virtual environment to a requirements.txt
file.
Installing Packages Using requirements.txt
The following command allows others to install the same packages specified in the requirements.txt
file:
pip install -r requirements.txt
This command automatically installs all the packages listed in the requirements.txt
file.
Important Notes When Using pip
Here are some important points to keep in mind when using pip:
- Check Virtual Environment Activation: Always ensure that the virtual environment is activated before installing packages. Installing packages globally by mistake can affect the entire system.
- Manage the requirements.txt File: As the project progresses, unnecessary packages may accumulate. It is a good practice to periodically update the
requirements.txt
file and remove any unnecessary packages. - Compatibility Issues: Be cautious of dependency conflicts between packages. If a specific version is required, make sure to specify it when installing the package.
Summary
A Python virtual environment is an essential tool for preventing library conflicts between different projects and for creating reproducible development environments. By utilizing pip and venv, you can easily create and manage virtual environments, and the requirements.txt
file allows you to systematically manage project packages.
By effectively using virtual environments, you can reduce confusion between projects and enjoy a better development experience. Therefore, every Python developer should understand the importance of virtual environments and actively utilize them.