Python min()
function is a built-in function that allows you to quickly find the minimum value in a given set of data. In this post, we will explore various ways to use the min()
function, along with examples and key points to keep in mind.
Table of Contents
Basic Concept of Python min() Function
The min()
function returns the smallest value among the given inputs. These inputs can be numbers, strings, lists, or other types of data. Python’s flexibility in handling data types makes it easy to find the minimum value across various data structures.
print(min(3, 1, 4, 1, 5))
As shown above, the min()
function returns the smallest value, which is 1, among the numbers 3, 1, 4, and 5.

Finding the Minimum Value in a List
A list is one of the most commonly used data structures in Python. The min()
function can be used to find the smallest element within a list.
numbers = [10, 20, 5, 30, 15]
print(min(numbers))
As seen in the example below, the minimum value in the list numbers
is 5. This function is very useful when dealing with a range of values in a list.

Finding the Minimum Value in a String
The min()
function can also be applied to strings. In the case of strings, the function compares the Unicode values of the characters and returns the smallest one.
text = "hello"
print(min(text))
The minimum value in a string is determined by the alphabetical order of the characters, and in this case, ‘e’ is considered the smallest character.
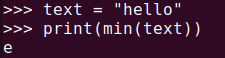
Important Notes When Handling Strings
When using min()
on a string, remember that the comparison is based on the Unicode values of each character. Therefore, uppercase letters, lowercase letters, and numbers are all compared. For instance, in the string “hello365”, ‘3’ is considered the smallest value.
Pythonmixed_text = "hello365"
print(min(mixed_text))
As shown below, when a string contains numbers, the numerical value ‘3’ is smaller than the letters.
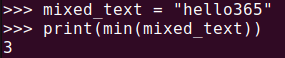
Finding the Minimum Value from Multiple Arguments
The min()
function can accept multiple arguments. By separating each argument with a comma, the function will return the minimum value among them.
print(min(3, 8, 1, 9, 6))
Finding the Minimum Value in a Dictionary
When dealing with dictionaries, the min()
function will return the minimum key by default. If you want to find the minimum value based on the dictionary’s values, additional methods are needed.
my_dict = {'a': 5, 'b': 3, 'c': 8}
print(min(my_dict))

To find the minimum value based on the values rather than the keys, you can use the values()
method along with min()
.
print(min(my_dict.values()))

Using the Key Parameter
The min()
function allows the use of the key
parameter to apply more complex conditions. For example, when the list elements are tuples, and you want to find the minimum value based on the second element of each tuple, you can use the key
parameter.
items = [(1, 'banana'), (3, 'apple'), (2, 'cherry')]
print(min(items, key=lambda x: x[1]))
In this example, the second element (string) of each tuple is used to find the minimum value. Using the key
parameter enables more flexible data processing.

Handling None Values
The min()
function throws a “TypeError: ‘<‘ not supported between instances of ‘NoneType’ and ‘int'” when trying to compare None
values, as shown below. This means None
cannot be compared with integer values.

If a list contains None
values, it is necessary to filter out or remove None
values beforehand.
numbers_with_none = [90, None, 8, 27]
filtered_numbers = [x for x in numbers_with_none if x is not None]
print(min(filtered_numbers))
By filtering out None
values, the min()
function works correctly as shown below.

Precautions
While the min()
function appears simple, its behavior can vary depending on the data types involved.
- Case Sensitivity in Strings: Unicode values of uppercase and lowercase letters differ, so the results may not always be as expected.
- Error with None Values: If
None
values are present in the list, an error will occur, and filtering is required. - Defining Functions with the Key Parameter: When dealing with complex data, make sure to define clear comparison criteria using the
key
parameter.
Summary
Python’s min()
function is a powerful tool that can easily find the minimum value across various data types such as numbers, strings, lists, and dictionaries. With the help of the key
parameter, it can handle more complex conditions and deliver flexible results. However, it’s important to be mindful of issues like case sensitivity in strings and handling of None
values. By understanding the appropriate usage of the min()
function, you can save time and effort in data processing tasks.